I think this will be easiest if you put your icons in a custom font and use a single label for all the text and icons. Here's my result with this approach:
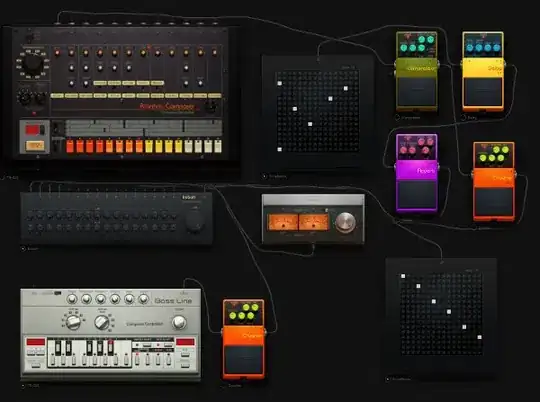
I used http://fontello.com/ to create a custom font with a record player, a pair of champagne glasses, and a clock. I put it in a file named appfont.ttf
.
Here's how I load the font:
let appFontURL = NSBundle.mainBundle().URLForResource("appfont", withExtension: "ttf")!
let appFontData = NSData(contentsOfURL: appFontURL)!
let appFontDescriptor = CTFontManagerCreateFontDescriptorFromData(appFontData)!
iOS has a default “cascade list” it uses to find glyphs for code points not in the font, but it's easy to override if you want to choose your own fallback font for the plain text. I used the Papyrus font to demonstrate:
let baseFontDescriptor = CTFontDescriptorCreateWithNameAndSize("Papyrus", 18)
let fontAttributes: [NSString:AnyObject] = [
kCTFontCascadeListAttribute : [ baseFontDescriptor ]
]
let fontDescriptor = CTFontDescriptorCreateCopyWithAttributes(appFontDescriptor, fontAttributes)
let font = CTFontCreateWithFontDescriptor(fontDescriptor, 18, nil) as UIFont
Next, I assemble all the text into one big string. You can see here how easy it is to replace different parts of the final string:
let recordPlayer = "\u{E802}"
let champagneGlasses = "\u{E801}"
let clock = "\u{E800}"
let nonBreakingSpace = "\u{00A0}"
let music1 = "Electro, Disco, 80's"
let music2 = "Jazz, Latino, Rock and roll, Electro, Beat Music"
let occasion = "After Office"
let time = "9:00 PM - 2:00 AM"
let text1 = "\(recordPlayer) \(music1) \(champagneGlasses)\(nonBreakingSpace)\(occasion)\n\(clock) \(time)"
let text2 = "\(recordPlayer) \(music2) \(champagneGlasses)\(nonBreakingSpace)\(occasion)\n\(clock) \(time)"
let text = text1 + "\n\n\n" + text2
I create a paragraph style to indent wrapped lines by 25 points:
let paragraphStyle = NSMutableParagraphStyle()
paragraphStyle.headIndent = 25
Now I can create the NSAttributedString
that applies the custom font and the paragraph style to the plain text:
let richText = NSAttributedString(string: text, attributes: [
NSFontAttributeName: font,
NSParagraphStyleAttributeName: paragraphStyle
])
At this point you would set label.attributedText = richText
, where label
is an outlet connected to the label in your storyboard. I'm doing this all in a playground, so to see how it looks, I create a label and size it to fit:
let label = UILabel(frame: CGRectMake(0, 0, 320, 0))
label.backgroundColor = UIColor.whiteColor()
label.attributedText = richText
label.numberOfLines = 0
label.bounds.size.height = label.sizeThatFits(CGSizeMake(label.bounds.size.width, CGFloat.infinity)).height
Finally, I draw the label into an image to see it:
UIGraphicsBeginImageContextWithOptions(label.bounds.size, true, 1)
label.drawViewHierarchyInRect(label.bounds, afterScreenUpdates: true)
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
image
You saw the result at the top of my answer. I've posted all the code and the fontello config in this gist.