A related answer: Difference between NSString literals
In short, when writing @""
in the global scope of your program, it does the same as writing ""
: The literal string you specified will be embedded in your binary at compile time, and can thus not be deallocated during runtime. This is why you don't need release
or retain
.
The @
just tells the compiler to construct a NSString object from the C string literal. Mind you that this construction is very cheap and probably heavily optimized. You can follow the link and see this example:
NSString *str = @"My String";
NSLog(@"%@ (%p)", str, str);
NSString *str2 = [[NSString alloc] initWithString:@"My String"];
NSLog(@"%@ (%p)", str2, str2);
Producing this output:
2011-11-07 07:11:26.172 Craplet[5433:707] My String (0x100002268)
2011-11-07 07:11:26.174 Craplet[5433:707] My String (0x100002268)
Note the same memory addresses
EDIT:
I made some test myself, see this code:
static NSString *str0 = @"My String";
int main(int argc, const char * argv[]) {
NSLog(@"%@ (%p)", str0, str0);
NSString *str = @"My String";
NSLog(@"%@ (%p)", str, str);
NSString *str2 = [[NSString alloc] initWithString:@"My String"];
NSLog(@"%@ (%p)", str2, str2);
return 0;
}
Will produce this output:
2015-12-13 21:20:00.771 Test[6064:1195176] My String (0x100001030)
2015-12-13 21:20:00.772 Test[6064:1195176] My String (0x100001030)
2015-12-13 21:20:00.772 Test[6064:1195176] My String (0x100001030)
Also, when using the debugger you can see that the actual object being created when using literals are in fact __NSCFConstantString
objects.
I think the related concept to that is called Class Clusters
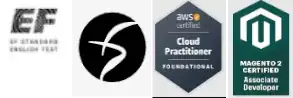