turns into a square
Means you don't have the supporting webfont
compiled (explained in a second).
You need to make sure you've compiled your assets, to allow the CSS
to both read and use the webfont
that's loaded by font-awesome
:
$ rake assets:precompile RAILS_ENV=production
$ git add .
$ git commit -a -m "Assets"
$ git push heroku master
We have several apps running font-awesome
in a Heroku production environment.
To do it, we used the font-awesome-rails
gem (this includes nice helpers):
<%= link_to fa-icon("users", text: "Title"), root_path, class:'navbar-brand' %>
We use the following SASS file:
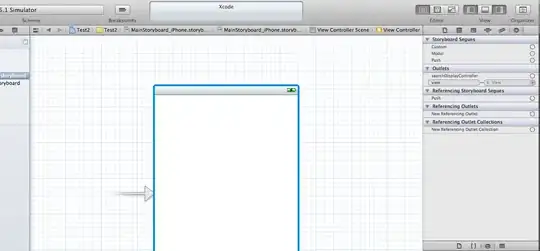
Doing this works for us -- make sure you precompile (mentioned above), and it should work for you.
Web Icons
There are several web icon "frameworks" (Ionic and Font-Awesome are the most popular). These basically work in the same way -- they have a custom font which they've compiled into a webfont.
This webfont is then made available in your app through a series of custom classes. These classes prepend a specific font "character" (icon) to the class with the :before
pseudoclass.
Thus, when you call fa-users
class, you're really getting this:
.fa-users:before {
content: "\f0c0";
}
The bottom line is that you need to make sure both the webfont
and the CSS stylesheets are precompiled properly before referencing either.