The MOV
(move) instruction transfer data between memory and registers or between registers. The MOV
instruction performs basic load data and store data operations between memory and the processor’s registers and data movement operations between registers.The MOV
instruction cannot move data from one memory location to another or from one segment register to
another segment register. Memory-to-memory moves are performed with the MOVS
(string move) instruction.
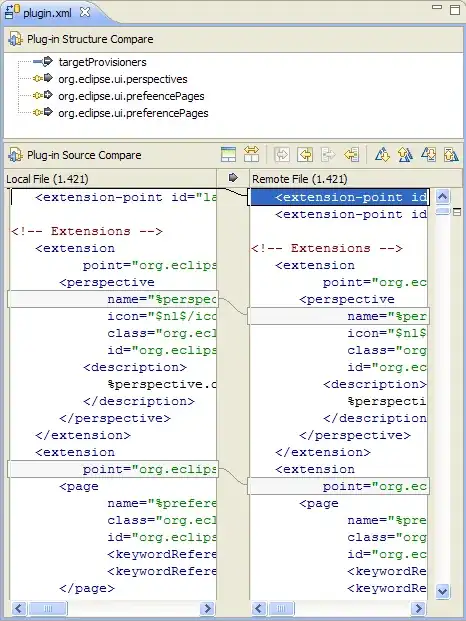
The LEA
(load effective address) instruction computes the effective address in memory (offset within a segment) of a source operand and places it in a general-purpose register. This instruction can interpret any of the processor’s
addressing modes and can perform any indexing or scaling that may be needed.
Intel 64 and IA-32 architectures Software Developer's manual
It's also highly useful as a 3-operand non-destructive add, doing e.g. ecx = eax + edx*4 - 15
NASM avoids this undesirable situation by having a much simpler syntax for memory references. The rule is simply that any access to the contents of a memory location requires square brackets around the address, and any access to the address of a variable doesn’t. So an instruction of the form mov ax, foo
will always refer to a compile−time constant, whether it’s an EQU
or the address of a variable; and to access the contents of the variable bar, you must code mov ax, [bar]
. This also means that NASM has no need for MASM’s OFFSET
keyword, since the MASM code mov ax, offset bar
means exactly the same thing as NASM’s mov ax,bar
.
Nasm documentation
From all the above you may see that that there are different ways to get the address of a variable in asm and these ways may and will differ in different assembly languages. To find an answer to your question it is good idea to check the documentation (assembly languages are usually well documented).
You should always remember in programming there are always several ways one particular problem can be solved.