In Swift or C# things get more complicated than they should be (IMHO) as you can not override the system font in a MKPointAnnotation
as you can not access the subview construction (or hierarchy) nor do some temporary ObjC magic on the system font calls.
- If someone does know how please tell me ;-)
I normally hide all this in a subclass'd MKMapView that uses completely custom drawn Annotation views instead of MKPointAnnotation
, but this way is easiest to follow.
So I assign a custom delegate to the GetViewForAnnotation
(a MKMapViewDelegate
) property of the MKMapView before starting to add annotations.
Example Setup/Call:
mapView.GetViewForAnnotation = myViewForAnnotation;
mapView.AddAnnotations (new MKPointAnnotation (){
Title="МУРАКАМИ В ТРЦ «ОКЕАН ПЛАЗА»",
Subtitle ="Пн - Пт 10.00 -00.00" +
" Cб.- Вс 10.00 - 00.00",
Coordinate = new CLLocationCoordinate2D (50.412300, 30.522756)
});
Custom GetViewForAnnotation (a MKMapViewDelegate):
This is where you assign the custom font for any new MKPointAnnotation
objects that need to be created but we are actually creating custom MKAnnotationView
's
public MyAnnotationView myViewForAnnotation(MKMapView mapView, IMKAnnotation id)
{
if (id is MKPointAnnotation) {
MyAnnotationView view = (MyAnnotationView)mapView.DequeueReusableAnnotation ("myCustomView");
if (view == null) {
view = new MyAnnotationView (id, "myCustomView", UIFont.FromName ("Chalkboard SE", 16f));
} else {
view.Annotation = id;
}
view.Selected = true;
return view;
}
return null;
}
Custom MyAnnotationView (MKAnnotationView subclass):
This stores the custom font that is passed via the constructor and handles the font assign on any UILabel
s that exist in its subviews:
using System;
using MapKit;
using UIKit;
namespace mkmapview
{
public class MyAnnotationView : MKAnnotationView // or MKPointAnnotation
{
UIFont _font;
public MyAnnotationView (IMKAnnotation annotation, string reuseIdentifier, UIFont font) : base (annotation, reuseIdentifier)
{
_font = font;
CanShowCallout = true;
Image = UIImage.FromFile ("Images/MapPin.png");
}
void searchViewHierarchy (UIView currentView)
{
// short-circuit
if (currentView.Subviews == null || currentView.Subviews.Length == 0) {
return;
}
foreach (UIView subView in currentView.Subviews) {
if (subView is UILabel) {
(subView as UILabel).Font = _font;
} else {
searchViewHierarchy (subView);
}
}
}
public override void LayoutSubviews ()
{
if (!Selected)
return;
base.LayoutSubviews ();
foreach (UIView view in Subviews) {
Console.WriteLine (view);
searchViewHierarchy (view);
}
}
}
}
System Font / Default size:
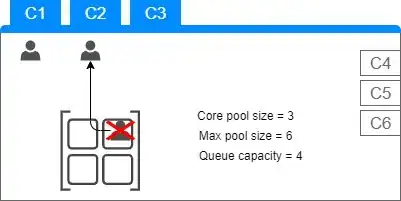
System Font / 10 point:

Custom Font (Chalkboard) / 10 point):
