Again, much better to avoid using null
layouts and setBounds(...)
. While null
layouts and setBounds()
might seem to Swing newbies like the easiest and best way to create complex GUI's, the more Swing GUI'S you create the more serious difficulties you will run into when using them. They won't re-size your components when the GUI resizes, they are a royal witch to enhance or maintain, they fail completely when placed in scrollpanes, they look gawd-awful when viewed on all platforms or screen resolutions that are different from the original one.
For example, the following code creates this GUI:
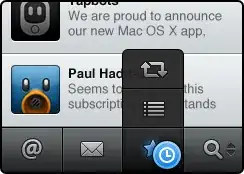
This uses a GridLayout to place a JPanel in the right hand side of another JPanel. If I wanted to add more components in different relative locations, it would be easy to do by simply nesting JPanels, each using its own layout.
import java.awt.Color;
import java.awt.Dimension;
import java.awt.GridLayout;
import javax.swing.*;
public class WorkWithLayouts extends JPanel {
private static final long serialVersionUID = 1L;
private static final int PREF_W = 400;
private static final int PREF_H = 315;
private static final String BG = "#ffffff";
public WorkWithLayouts() {
JPanel mostInner = new JPanel();
mostInner.setForeground(Color.black);
mostInner.setOpaque(false); // if you want the backing jpanel's background to show through
// add title temporarily just to show where mostInner panel is
mostInner.setBorder(BorderFactory.createTitledBorder("most inner")); // TODO: delete this
JLabel jltxt = new JLabel();
jltxt.setText("Test");
mostInner.add(jltxt);
setBackground(Color.decode(BG));
setLayout(new GridLayout(1, 2));
add(new JLabel()); // empty label
add(mostInner);
}
@Override
public Dimension getPreferredSize() {
if (isPreferredSizeSet()) {
return super.getPreferredSize();
} else {
return new Dimension(PREF_W, PREF_H);
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
createAndShowGui();
});
}
private static void createAndShowGui() {
WorkWithLayouts mainPanel = new WorkWithLayouts();
JFrame frame = new JFrame("Work With Layouts");
frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
frame.add(mainPanel);
frame.pack();
frame.setLocationByPlatform(true);
frame.setVisible(true);
}
}