You can try this script rename_files.py
. you should place this script inside the folder where all your files
are located.
Also note:
os.rename(src, dst)
Rename the file or directory src to dst. If dst is a directory,
OSError will be raised. On Unix, if dst exists and is a file, it will
be replaced silently if the user has permission. The operation may
fail on some Unix flavors if src and dst are on different filesystems.
If successful, the renaming will be an atomic operation (this is a
POSIX requirement). On Windows, if dst already exists, OSError will be
raised even if it is a file; there may be no way to implement an atomic rename when dst names an existing file.
import os
list_of_files = os.listdir(os.getcwd())
n = 1
for filename in list_of_files:
if not filename.endswith(".py"):
os.rename(filename, str(n))
n += 1
Before running this script you can see the file_names
and directory
.
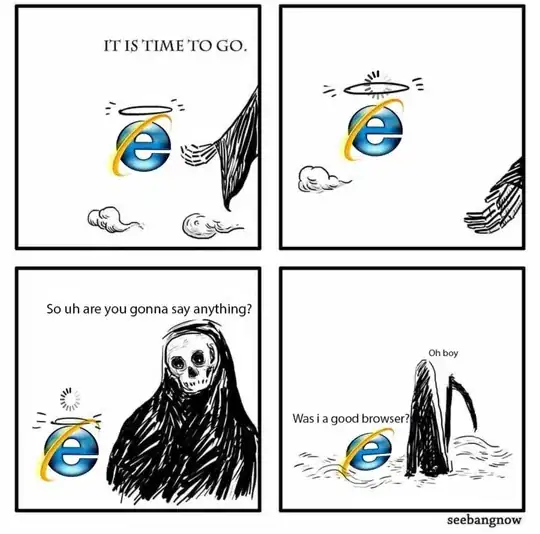
After running this script. I hope this is helpful
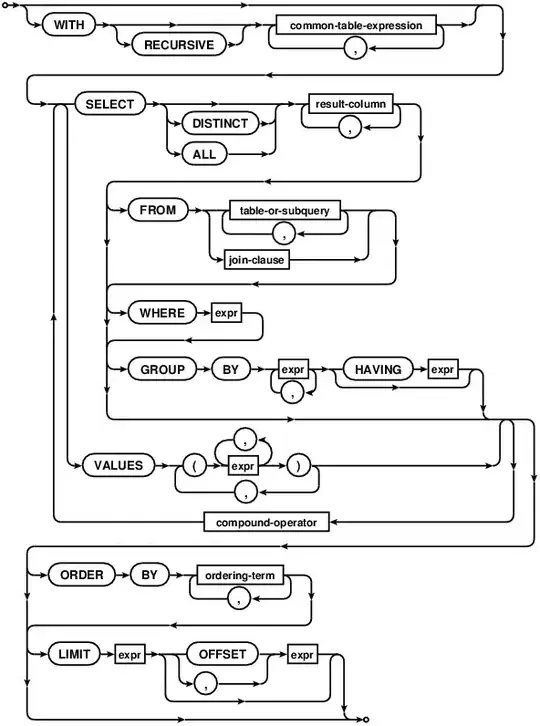