Imagine an extensible ring made of rubber. Uniformly distribute several points on this ring. When it has small radius, the density of points is high. But when you try to increase the radius or ring by stretching it the density of points on the ring would fall. That is the main problem in your algorithm.
Instead, try the following approach:
1. Draw a biggest possible circle on a square piece of paper
2. Put a point randomly on the paper
3. If it's outside a circle reject point and go to step 2
4. Repeat steps 2-3 until you get the necessary density
The above algorithm is implemented using complex numbers with loops:
n = 848;
Rc = 30;
Xc = 0;
Yc = 0;
x = 2*(rand(1,n) + 1i*rand(1,n)) - 1 - 1i;
for i = 1:length(x)
while abs(x(i)-Xc-1i*Yc) > 1
x(i) = 2*(rand + 1i*rand) - 1 - 1i;
end
end
x = Rc * x + Xc + 1i*Yc;
plot(x,'.');
t = linspace(0,2*pi);
hold on
plot(Rc*cos(t)+Xc,Rc*sin(t)+Yc)
axis equal;
hold off;
The 5th line generates 848 random points with x
and y
coordinates between -1 and 1. Then, the loop checks each random point for being inside unit circle and if not, replaces it with another random point. Finally, we multiply your random points by 30 and shift them along X and Y axes (zero shift in your case). This you get as a result:
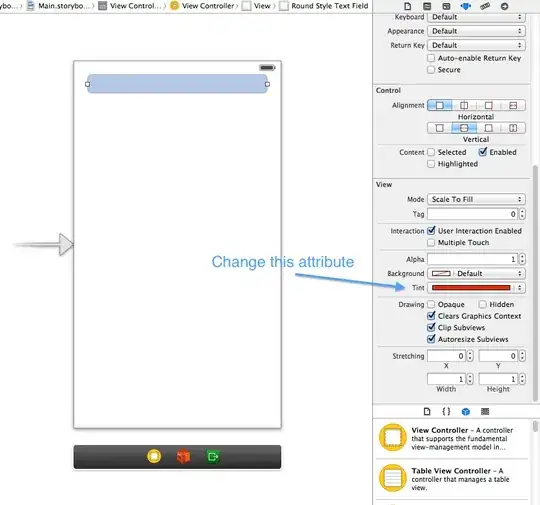
UPD to OP's comment: it's not exactly 0.3 per m^2 for any m^2, but it's close to this number. If you want this to be checked using squares, then you would define it as some range along x
and some range along y
. Then you can use:
xmin = 5;
xmax = 20;
ymin = 5;
ymax = 20;
if all(abs([xmin + ymin*1i, xmin + ymax*1i, xmax + ymin*1i, xmax + ymax*1i]) < Rc)
density = nnz(real(x) > xmin & real(x) < xmax & imag(x) > ymin & imag(x) < ymax)/(xmax-xmin)/(ymax-ymin)
else
error('Square is outside circle')
end
density =
0.2800
So, you manually define your x and y limits that form your test square. Then, the square are checked to be inside circle and if it is, the density is checked.