If JS was fully supported, you could use a DOM-based solution.
var html = "<font color=\"#FF0202\">NOT THIS ONE</font><font color=\"#FF0101\">\n Data which is want to fetch\n</font>";
var faketag = document.createElement('faketag');
faketag.innerHTML = html;
var arr = [];
[].forEach.call(faketag.getElementsByTagName("font"), function(v,i,a) {
if (v.hasAttributes() == true) {
for (var o = 0; o < v.attributes.length; o++) {
var attrib = v.attributes[o];
if (attrib.name === "color" && attrib.value === "#FF0101") {
arr.push(v.innerText.replace(/^\s+|\s+$/g, ""));
}
}
}
});
document.body.innerHTML = JSON.stringify(arr);
However, acc. to the GAS reference:
However, because Apps Script code runs on Google's servers (not client-side, except for HTML-service pages), browser-based features like DOM manipulation or the Window API are not available.
You may try obtaining the inner text of <font color="#FF0101">
tags with a regex:
function myFunction() {
var doc = DocumentApp.getActiveDocument();
var paras = doc.getParagraphs();
var MyRegex = new RegExp('<font\\b[^<]*\\s+color="#FF0101"[^<]*>([\\s\\S]*?)</font>','ig');
for (i=0; i<paras.length; ++i) {
while (match = MyRegex.exec(paras[i].getText()))
{
Logger.log(match[1]);
}
}
}
Result against <font color="#FF0202">NOT THIS ONE</font><font color="#FF0101"> Data which is want to fetch</font>
:
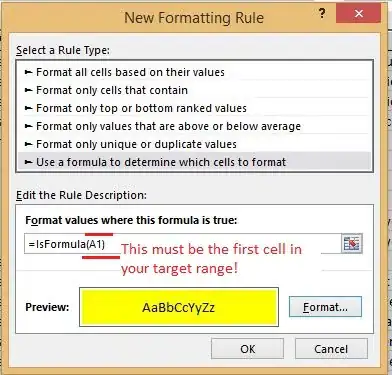
Regex matches any font
tag that have color
attribute with the value of #FF0101
inside double quotation marks. Mind that regexps are not reliable when parsing HTML! A better regex for this task is
<font\\b[^<]*\\s+color="#FF0101"[^<]*>([^<]*(?:<(?!/font>)[^<]*)*)</font>
In case your HTML data spans across several paragraphs, use
function myFunction() {
var doc = DocumentApp.getActiveDocument();
var text = doc.getBody().getText();
var MyRegex = new RegExp('<font\\b[^<]*\\s+color="#FF0101"[^<]*>([\\s\\S]*?)</font>','ig');
while (match = MyRegex.exec(text))
{
Logger.log(match[1]);
}
}
With this input:
<font color="#FF0202">NOT THIS ONE</font>
<font color="#FF0101">
Data which is want to fetch
</font>
Result is:
