If you just want to create a control in an UWP app like your second picture, you can do it like below:
<Button Background="Transparent" Click="OnClick" HorizontalAlignment="Center">
<RelativePanel>
<TextBlock FontFamily="Segoe MDL2 Assets" FontSize="30" Text=""/>
<Border Background="Red" RelativePanel.AlignBottomWithPanel="True" RelativePanel.AlignRightWithPanel="True">
<TextBlock x:Name="count" Foreground="White" Text="{x:Bind tb.Text,Mode=OneWay}" FontSize="12" />
</Border>
</RelativePanel>
</Button>
<TextBox VerticalAlignment="Bottom" x:Name="tb" />
In this sample, I bind the Text of the TextBlock
named "count" to a TextBox
, so we can change this value.
But we can create it as an UserControl, so that we can use it repeatable. And we can use a DependencyProperty to expose the property we want to use. For example here:
<UserControl
x:Class="BadgeCountApp.MyUserControl"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:BadgeCountApp"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Button Background="Transparent" HorizontalAlignment="Center">
<RelativePanel>
<TextBlock FontFamily="Segoe MDL2 Assets" FontSize="30" x:Name="text" Text="{x:Bind SymbText,Mode=OneWay}"/>
<Border Background="Red" RelativePanel.AlignBottomWithPanel="True" RelativePanel.AlignRightWithPanel="True">
<TextBlock x:Name="count" Foreground="White" FontSize="12" Text="{x:Bind SymbCount,Mode=OneWay}" Visibility="Collapsed"/>
</Border>
</RelativePanel>
</Button>
</UserControl>
code behind:
public sealed partial class MyUserControl : UserControl
{
public MyUserControl()
{
this.InitializeComponent();
}
public static readonly DependencyProperty SymbTextProperty = DependencyProperty.Register("SymbText", typeof(string), typeof(MyUserControl), new PropertyMetadata(string.Empty));
public static readonly DependencyProperty SymbCountProperty = DependencyProperty.Register("SymbCount", typeof(int), typeof(MyUserControl), new PropertyMetadata(0, new PropertyChangedCallback(ChangedCallback)));
private static void ChangedCallback(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
MyUserControl muc = (MyUserControl)d;
int value = (int)e.NewValue;
if (value == 0)
{
muc.count.Visibility = Visibility.Collapsed;
}
else
{
muc.count.Visibility = Visibility.Visible;
}
}
public string SymbText
{
get { return (string)GetValue(SymbTextProperty); }
set { SetValue(SymbTextProperty, value); }
}
public int SymbCount
{
get{ return (int)GetValue(SymbCountProperty);}
set{ SetValue(SymbCountProperty, value);}
}
}
Now you can use this control directly in other place like this:
<local:MyUserControl x:Name="user" SymbText="" Tapped="OnTapped"/>
code behind:
private void OnTapped(object sender, TappedRoutedEventArgs e)
{
if (user.SymbCount == 0)
user.SymbCount = 3;
else
user.SymbCount = 0;
}
The following is a screenshot of this UserControl
:
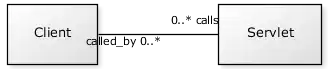