You don't need setSupportActionBar
for your cardviews with toolbars until you would need to set some ActionBar specific actions up/back navigation actions.
Take a look at this fine example (link to whole page below):
Thanks Gabriele for all the help. Here is working code:
Activity :
public class MainActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_mai);
Toolbar toolbar = (Toolbar) findViewById(R.id.card_toolbar);
toolbar.setTitle("Card Toolbar");
if (toolbar != null) {
// inflate your menu
toolbar.inflateMenu(R.menu.main);
toolbar.setOnMenuItemClickListener(new Toolbar.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem menuItem) {
return true;
}
});
}
Toolbar maintoolbar = (Toolbar) findViewById(R.id.toolbar_main);
if (toolbar != null) {
// inflate your menu
maintoolbar.inflateMenu(R.menu.main);
maintoolbar.setOnMenuItemClickListener(new Toolbar.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem menuItem) {
return true;
}
});
}
}
}
Layout XML File:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar_main"
android:layout_width="match_parent"
android:layout_height="@dimen/action_bar_size_x2"
android:background="@android:color/holo_orange_dark"
android:minHeight="?attr/actionBarSize" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@+id/toolbar_main"
android:layout_marginLeft="15dp"
android:layout_marginRight="15dp"
android:layout_marginTop="@dimen/action_bar_size"
android:orientation="vertical" >
<android.support.v7.widget.CardView
android:layout_width="match_parent"
android:layout_height="match_parent" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<android.support.v7.widget.Toolbar
android:id="@+id/card_toolbar"
android:layout_width="match_parent"
android:layout_height="@dimen/action_bar_size"
android:background="@android:color/white" />
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="@android:color/darker_gray" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:text="@string/app_name"
android:textSize="24sp" />
</LinearLayout>
</android.support.v7.widget.CardView>
</LinearLayout>
</RelativeLayout>
Make sure your activity theme is extending Theme.AppCompat.Light.NoActionBar
.
Here is what it will look like:
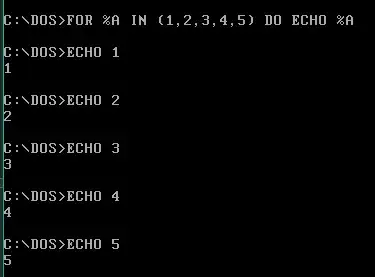
Few things to note:
- If you are using card elevation then you need to altar the margin top so that your card aligns with the main toolbar
- I am still seeing 1-2 pixel margin between bottom of main toolbar and card toolbar. Not sure about what to do in this case. For now, i
am aligning it manually.
From: How to create a card toolbar using appcompat v7
Notice that author of this post hadn't used it at all, so I'm pretty sure you would also don't need that for implementation your idea
Hope it help