I'm not sure I understand the entire problem or what it is you ultimately want to achieve, but, lets have a look at some of the issues you are having to start with...
First, you create a JScrollPane
which wraps a JTextArea
...
JScrollPane scroll = new JScrollPane(ta);
Okay, this is good.
Next, using a KeyListener
(which is never registered in you code snippet), you either add the JScrollPane
OR the JTextArea
to the screen
if(e.getKeyCode() == KeyEvent.VK_CONTROL){
if(s == false){
s = true;
remove(scroll);
ta.setLineWrap(true);
add(ta);
repaint();
}else{
s = false;
ta.setLineWrap(false);
remove(ta);
add(scroll);
repaint();
}
}
There are actually three problems with this...
- When adding a component to a container, it is first removed from it's previous parent, in this case, when you add your
JTextArea
to the frame, you remove it from the JScrollPane
, but you never reapply it, so your JScrollPane
will be empty the next time you add it
- When updating a UI dynamically like this, you need to call
revalidate
followed by repaint
to ensure that the layout is update
KeyListener
is notorious for not working, this is due to the fact that the component that it is registered to must be focusable AND have focus before it will trigger, a better solution would be to use the Key Bindings API
But how to fix it? Normally, when switching between components/views, I'd recommend a CardLayout
, but to be honest, I'm not sure why you want to remove the JScrollPane
, so instead, I've just set it up to change the lineWrap
state via the Control key instead
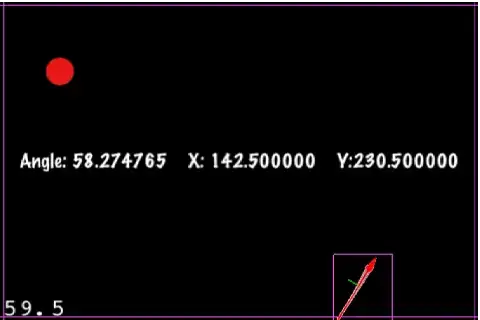
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.KeyEvent;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.io.Reader;
import javax.swing.AbstractAction;
import javax.swing.ActionMap;
import javax.swing.InputMap;
import static javax.swing.JComponent.WHEN_IN_FOCUSED_WINDOW;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.KeyStroke;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class Switcher {
public static void main(String[] args) {
new Switcher();
}
public Switcher() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
ex.printStackTrace();
}
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class TestPane extends JPanel {
private JTextArea ta;
public TestPane() {
setLayout(new BorderLayout());
ta = new JTextArea(10, 20);
ta.setLineWrap(true);
add(new JScrollPane(ta));
try (Reader r = new FileReader(new File("Labyrinth.txt"))) {
ta.read(r, "Labyrinth");
} catch (IOException ex) {
ex.printStackTrace();
}
InputMap inputMap = getInputMap(WHEN_IN_FOCUSED_WINDOW);
ActionMap actionMap = getActionMap();
inputMap.put(KeyStroke.getKeyStroke(KeyEvent.VK_CONTROL, KeyEvent.CTRL_DOWN_MASK), "pressed.control");
actionMap.put("pressed.control", new AbstractAction() {
@Override
public void actionPerformed(ActionEvent e) {
ta.setLineWrap(!ta.getLineWrap());
}
});
}
}
}