You can use Microsoft Fakes (assuming you have, I think, Ultimate edition of Visual Studio).
https://msdn.microsoft.com/en-us/library/hh549175.aspx
This will allow you to fake out static methods :)
Add a Fakes assembly for System:
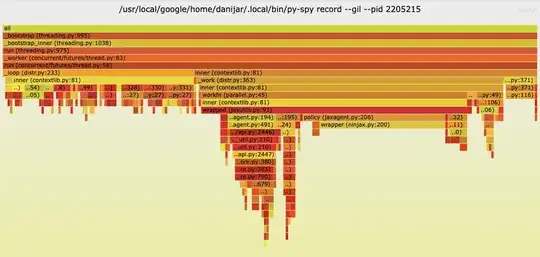
Open "Fakes → mscorlib.fakes" and edit it to look like this:
<Fakes xmlns="http://schemas.microsoft.com/fakes/2011/">
<Assembly Name="mscorlib" Version="4.0.0.0"/>
<ShimGeneration>
<Add FullName="System.Environment"/>
</ShimGeneration>
</Fakes>
Perform a build.
You can now write your unit test:
[TestMethod]
public void TestMethod1()
{
using (ShimsContext.Create())
{
System.Fakes.ShimEnvironment.OSVersionGet = () => new OperatingSystem(PlatformID.Win32Windows, new Version("99.99"));
Assert.AreEqual(Environment.OSVersion.Version.Major, 99);
}
}