The data you want is being populated by javascripts.
You would have to use a selenium webdriver
to extract the data.
If you want to check before hand if data is being populated using javascript, open a scrapy shell and try extracting the data as below.
scrapy shell 'http://www.lazada.vn/dien-thoai-may-tinh-bang/?ref=MT'
>>>response.xpath('//div[contains(@class,"product-card")]')
Output:
[]
Now, if you use the same Xpath in the browser and get a result as below:
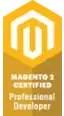
Then the data is populated using scripts and selenium would have to be used to get data.
Here is an example to extract data using selenium:
import scrapy
from selenium import webdriver
from scrapy.http import TextResponse
class ProductSpider(scrapy.Spider):
name = "product_spider"
allowed_domains = ['lazada.vn']
start_urls = ['http://www.lazada.vn/dien-thoai-may-tinh-bang/?ref=MT']
def __init__(self):
self.driver = webdriver.Firefox()
def parse(self, response):
self.driver.get(response.url)
page = TextResponse(response.url, body=self.driver.page_source, encoding='utf-8')
required_data = page.xpath('//div[contains(@class,"product-card")]').extract()
self.driver.close()
Here are some examples of "selenium spiders":
- Executing Javascript Submit form functions using scrapy in python
- Snipplr
- Scrapy with selenium
- Extract data from dynamic webpages