The idea of modelling, in simple terms, involves finding a mathematical representation of a physical phenomenon. In other words you need to "find" an equation that represents all the observable properties of the object in context, in your case a pendulum1.
All you need to describe a pendulum is its equation of motion. To find it you firstly try to qualify the motion, by observing it:
If you try to displace it (many times with a very small angle) from its equilibrium point, you will mostly observe few swings, along the same path, where its amplitude decreases and it is brought back to its stable equilibrium, as if by some kind of restoring force, in other words the pendulum performs regular and repeating motion, called periodic motion
Thus, you are looking for a mathematical function that can represent a periodic motion, as it turns out a great candidate for this role are the trigonometric functions: sin and cos, that have the needed property i.e. repeat themselves with a period T.
To find and quantify this restoring force you use Newton's Second Law, where after you express everything in terms of the very small angle theta << 1
(that allows using of the small-angle approximation: sin(theta) = theta
) of displacement theta
, you get the wanted equation of motion, which is represented by the following differential equation:
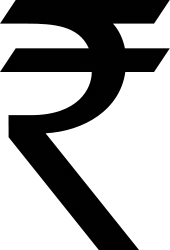
with a great surprise (and to the first order of approximation), when you solve the above equation you find:
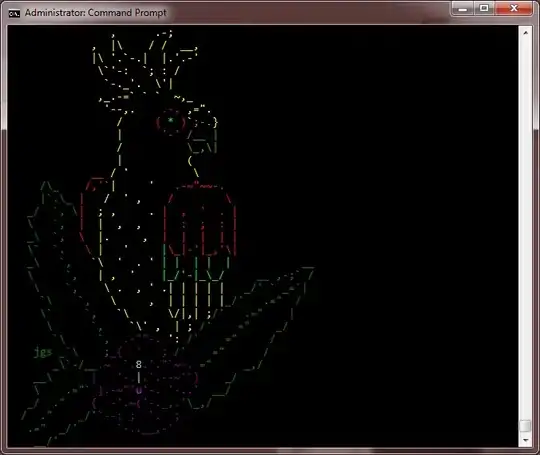
which approximately2 matches the predictions from your observations (with some random error (standard deviation), preferably not systematic), i.e. the motion of the pendulum is described by the cosine function, it has periodic motion with an amplitude equal to the initial displacement and a period equal to:
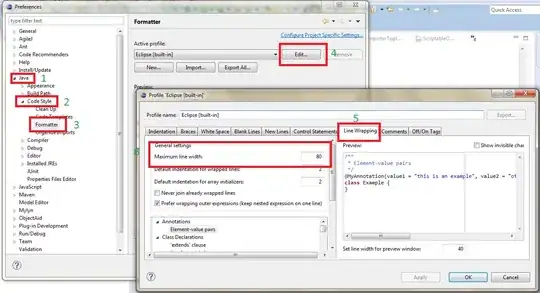
and here is the catch,
the above equation for theta
describes a pendulum that does not lose energy and its values repeat infinitely and that is why the loop in your program is an infinite loop harmonically oscillating pun intended with the same values!
If you want to observe a motion that slowly stops you need to add an additional damping force that will slowly subtract energy from the system. This damping force is represented by the following additional term in the equation of motion:
that again, can be expressed in terms of the angle theta
. Now, when you solve the equation of motion, the amplitude gets multiplied by:
where b
is the damping constant from which it depends how fast the motion will stop.
As a consequence of the above,
if you want to see something similar to the real pendulum you need to include this last exponential to your equation.
To do this just follow the great explanation offered by @Petr Stepanov, modifying thetaMax
to include the above exponent.
#include <stdio.h>
#include <math.h>
#define PI 3.14159
#define E 2.71828 // Euler's number
#define L 1 // length of the pendulum
#define g 9.80665 // gravity const
int main() {
double theta = 0.0; // initial value for angle
double omega = 0.2; // initial value for angular speed
double time = 0.0; // initial time
double dt = 0.01; // time step
double b = 0.5; // modified damping constant
double thetaMax = omega * omega * L / (2 * g);
while (theta < thetaMax) {
time = time + dt;
theta = thetaMax * ldexp(E, (-b) * time) * sin(omega * time);
printf("Deflection angle=%f, Angular frequency=%f, Time=%f\n", theta, omega, time);
}
return 0;
}
1. An object with mass m, hung on a fixed point with a non-elastic, "massless" thread with length l , that allows it to swing freely in a gravitational field, quantified by the gravitational acceleration constant g.
2. It should be noted that: "all models are wrong; the practical question is how wrong do they have to be to not be useful"