Assuming that your file is in the sdcard and that youll name it the same as your button this should work.
Also, in your first activity you can make a public variable(or with the extras that Hi I'm Frogatto mentioned. they really sound a better idea than mine ) that stores the name of the button clicked and then add it +".txt".
Ps: i took the time to make this just because of my curiosity c:
public class test extends Activity {
LinearLayout layout;
Button btnarr [] = new Button[50];
int counter = 0,check=0;
protected void onCreate(Bundle savedInstanceState) {
counter=0;
super.onCreate(savedInstanceState);
setContentView(R.layout.test);
layout = new LinearLayout(this);
layout.setLayoutParams(new ViewGroup.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.MATCH_PARENT));
layout.setOrientation(LinearLayout.VERTICAL);
File sdcard = Environment.getExternalStorageDirectory();
//Get the text file
File file = new File(sdcard,"yourbuttonname.txt");
//Read text from file
StringBuilder text = new StringBuilder();
try {
BufferedReader br = new BufferedReader(new FileReader(file));
String line;
while ((line = br.readLine()) != null) {
text.append(line);
btnarr[counter] = new Button(this);
btnarr[counter].setText(text.toString());
text = new StringBuilder();
counter++;
check++;
}
br.close();
}
catch (IOException e) {
e.printStackTrace();
}
doit();
}
public void doit()
{
counter = 0;
while(counter < check)
{
LinearLayout row = new LinearLayout(this);
row.setLayoutParams(new ViewGroup.LayoutParams(ViewGroup.LayoutParams.FILL_PARENT, ViewGroup.LayoutParams.WRAP_CONTENT));
btnarr[counter].setLayoutParams(new ViewGroup.LayoutParams(ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT));
row.addView(btnarr[counter]);
layout.addView(row);
counter++;
}
setContentView(layout);
}
}
test.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
</LinearLayout>
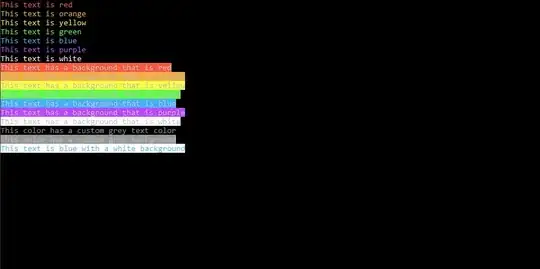
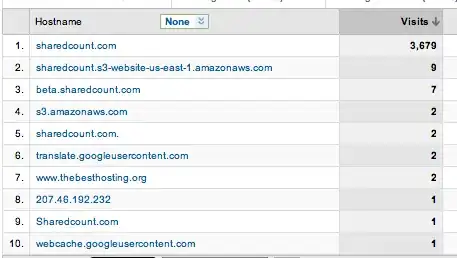