Using jQuery, you can use:
//Change window, for any other element you want to check. IE: document
$._data(window, "events");
You can check all jquery events attached to an element, so if someone did:
$(window).on("scroll", function(){ console.log("scroll"); });
You'll see something like this:
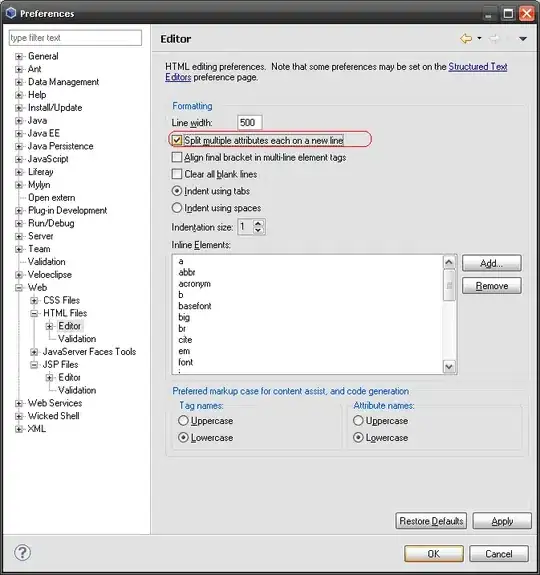
Using plain javascript, you can "intercept" the addEventListener
and attachEvent
to check all events listeners attached to window, or any element for that matter:
(function(){
function interceptListener(type, element){
if(typeof element[type] == "function"){
element[type] = (function(){
var original = this[type];
this.allListeners = this.allListeners || {};
return function() {
this.allListeners[arguments[0]] = arguments[0] in this.allListeners ? this.allListeners[arguments[0]] + 1 : 1;
return original.apply(this, arguments);
}
}.bind(element))();
}
}
interceptListener("attachEvent", window);
interceptListener("addEventListener", window);
})();
Place this snippet before any other scripts.
If you want to check if window.onscroll
was set, you can just test on window load if any other script set it:
if(typeof window.onscroll == "function")
console.log("onscroll function");
Or you can watch the property with: object watch polyfill
window.watch("onscroll", function(){
if(typeof this.onscroll == "function")
this.allListeners["onscroll"] = this.allListeners["onscroll"] + 1 : 1;
});
Then you can check how many listeners are attached to window with:
console.log(window.allListeners);
NOTE: as @JuanMendes pointed out: Be aware that this is an internal data structure that is undocumented and should not be modified
and should only be used for debugging.