Let's disregard performance (speed) and best practice for a bit.
eps(n)
is a command that returns the distance to the next larger double precision number from n
in MATLAB. So, eps(1) = 2.2204e-16
means that the first number after 1
is 1 + 2.2204e-16
. Similarly, eps(3000) = 4.5475e-13
. Now, let's look at the precision of you calculations:
n = 100;
A = rand(n);
inv_A_1 = inv(A);
inv_A_2 = A \ eye(n);
max(max(abs(inv_A_1-inv_A_2)))
ans =
1.6431e-14
eps(127) = 1.4211e-14
eps(128) = 2.8422e-14
For integers, the largest number you can use that has an accuracy higher than the max difference between your two matrices is 127.
Now, let's check how the accuracy when we try to recreate the identity matrix from the two inverse matrices.
error_1 = max(max(abs((A\eye(size(A))*A) - eye(size(A)))))
error_1 =
3.1114e-14
error_2 = max(max(abs((inv(A)*A) - eye(size(A)))))
error_2 =
2.3176e-14
The highest integer with a higher accuracy than the maximum difference between the two approaches is 255.
In summary, inv(A)
is more accurate, but once you start using the inverse matrices, they are for all intended purposes identical.
Now, let's have a look at the performance of the two approaches:
n = fix(logspace(1,3,40));
for i = 1:numel(n)
A = rand(round(n(i)));
t1(i) = timeit(@()inv(A));
t2(i) = timeit(@()A\eye(n(i)));
end
loglog(n,[t1;t2])
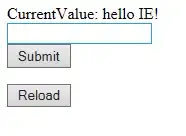
It appears that which of the two approaches is fastest is dependent on the matrix size. For instance, using inv
is slower for n = 255
, but faster for n = 256
.
In summary, choose approach based on what's important to you. For most intended purposes, the two approaches are identical.
Note that svd
and pinv
may be of interest if you're working with badly scaled matrices. If it's really really important you should consider the Symbolic toolbox.
I know you said that you "actually need the inverse", but I can't let this go unsaid: Using inv(A)*b
is never the best approach for solving a linear equation! I won't explain further as I think you know this already.