You can eliminate z
from the first two equations to give x=1
and the line of intersection of the first two planes z=7+2y
, and then solve with the remaining equation to give the point (1,-2,3)
. You can verify this with numpy.linalg.solve
:
In [11]: M = np.array([[4., -2., 1.], [-2., 4., -2.], [1., -2., 4.]])
In [12]: b = np.array([11., -16., 17.])
In [13]: np.linalg.solve(M, b)
Out[13]: array([ 1., -2., 3.])
In Matplotlib, the planes can be plotted with plot_surface
, the line of intersection of the first two (in blue) with plot
and a marker used for the point at which the third plane (in green) intersects the line.
Make the planes a bit transparent with alpha=0.5
and because the planes re flat, there's no need for marker lines to tile the surfaces (you can set the row strides and column strides to something large):
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x, y = np.linspace(-8,8,100), np.linspace(-8,8,100)
X, Y = np.meshgrid(x,y)
Z1 = 11 - 4*X + 2*Y
Z2 = (16 - 2*X + 4*Y) / 2
Z3 = (17 - X + 2*Y) / 4
ax.plot_surface(X,Y,Z1, alpha=0.5, rstride=100, cstride=100)
ax.plot_surface(X,Y,Z2, alpha=0.5, rstride=100, cstride=100)
ax.plot((1,1),(-8,8),(-9,23), lw=2, c='b')
ax.plot_surface(X,Y,Z3, alpha=0.5, facecolors='g', rstride=100, cstride=100)
ax.plot((1,),(-2,),(3,), lw=2, c='k', marker='o')
plt.show()
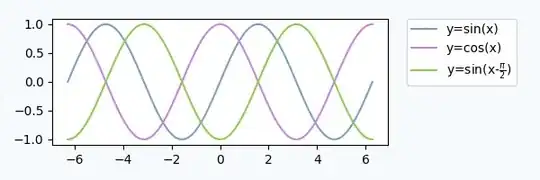