Ahmad's solution is great if you don't mind changing the values. But what if you want to keep the original values and have the inverted versions?
Let's say you have a folder named Converters and in it you create the two following IValueConverter
classes:
The base class converting a System.Windows.Media.Color
to a System.Windows.Media.SolidColorBrush
:
using System;
using System.Globalization;
using System.Windows.Data;
using System.Windows.Media;
namespace WPFTest.Converters
{
public class ColorToBrush : IValueConverter
{
public virtual object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
return value is Color ? new SolidColorBrush((Color)value) : Brushes.Black;
}
public virtual object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
}
An inheriting class to invert the color - using any of these methods - then convert it to a brush:
using System;
using System.Globalization;
using System.Windows.Media;
namespace WPFTest.Converters
{
public class InvertColorToBrush : ColorToBrush
{
public override object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (value is Color)
{
Color color = (Color)value;
int iCol = ((color.A << 24) | (color.R << 16) | (color.G << 8) | color.B) ^ 0xffffff;
Color inverted = Color.FromArgb((byte)(iCol >> 24),
(byte)(iCol >> 16),
(byte)(iCol >> 8),
(byte)(iCol));
return base.Convert(inverted, targetType, parameter, culture);
}
else
{
return Brushes.Black;
}
}
public override object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
}
Note: Gray-scale color's inversions are less dramatic, so for this example I added:
<Color x:Key="Blue">#0000ff</Color>
<Color x:Key="Yellow">#ffff00</Color>
Then in xaml you add your reference:
xmlns:converters="clr-namespace:WPFTest.Converters"
Declare your keys:
<converters:ColorToBrush x:Key="BrushColor" />
<converters:InvertColorToBrush x:Key="BrushInvertColor" />
And usage:
<Label
Content="COLOR TEST"
Background="{Binding Converter={StaticResource BrushColor}, Mode=OneWay, Source={StaticResource Blue}}"
Foreground="{Binding Converter={StaticResource BrushColor}, Mode=OneWay, Source={StaticResource Yellow}}"/>
<Label
Content="COLOR INVERT TEST"
Background="{Binding Converter={StaticResource BrushInvertColor}, Mode=OneWay, Source={StaticResource Blue}}"
Foreground="{Binding Converter={StaticResource BrushInvertColor}, Mode=OneWay, Source={StaticResource Yellow}}"/>
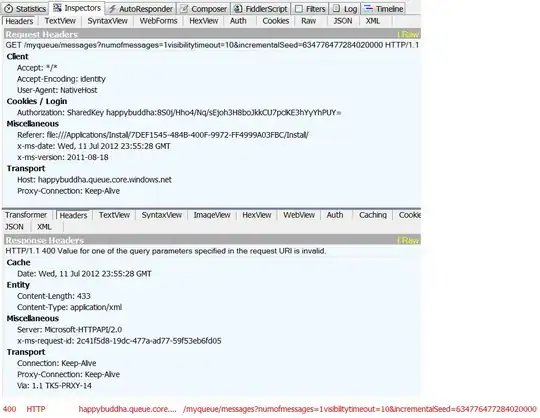