Here's one way to approach it in Oracle as I answered in this post: Oracle 11gR2: split string with multiple delimiters(add). Hopefully you can apply the logic to your RDBMS. Note that this answer doesn't just get the value from the string, but attempts to parse the string and return values so they can be processed like rows in a query's result set. This may be overkill for your scenario. At any rate, it's just one way to look at it.
-- Original data with multiple delimiters and a NULL element for testing.
with orig_data(str) as (
select 'DeliveryMethod+NON;Installation_Method+NoInstallation;;Services_Reference_ID+100118547,44444,33333;Service_ID+2222' from dual
),
--Split on first delimiter (semi-colon)
Parsed_data(rec) as (
select regexp_substr(str, '(.*?)(;|$)', 1, LEVEL, NULL, 1)
from orig_data
CONNECT BY LEVEL <= REGEXP_COUNT(str, ';') + 1
)
-- For testing-shows records based on 1st level delimiter
--select rec from parsed_data;
-- Split the record into columns
select regexp_replace(rec, '^(.*)\+.*', '\1') col1,
regexp_replace(rec, '^.*\+(.*)', '\1') col2
from Parsed_data;
Result:
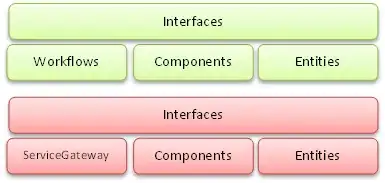
To specifically answer your question, in order to get a value based on a key, change the last query to this in order to get the value where the key is 'Service_ID':
select value
from (
select regexp_replace(rec, '^(.*)\+.*', '\1') key,
regexp_replace(rec, '^.*\+(.*)', '\1') value
from Parsed_data )
where key = 'Service_ID';
Result:

Or to just extract it out of the string using a regular expression:
with orig_data(str) as (
select 'Service_ID+2222;DeliveryMethod+NON;Installation_Method+NoInstallation;;Services_Reference_ID+100118547,44444,33333' from dual
)
select regexp_substr(str, '(.*?)Service_ID\+(.+?)(;|$)', 1, 1, NULL, 2) value
from orig_data;