However, I would like to show the same progress indication through the
whole app. Consider e.g. another activity that looks like a form with
submit button. There is neither a ListView nor a SwipeRefreshLayout in
this form activity. Some progress indication should be displayed while
the submitted data are transferred to the server. I want to show a
progress bar with the same animation like in SwipeRefreshLayout.
yes you can use SwipeRefreshLayout
to show like ProgressDialog
when button click. check below.
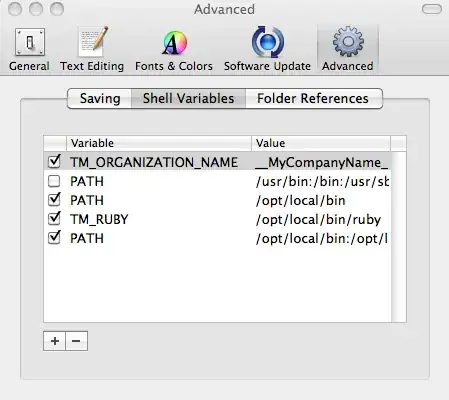
i have added SwipeRefreshLayout
inside my layout file still there is not ListView
or ScrollView
. just like below
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
app:layout_behavior="@string/appbar_scrolling_view_behavior"
tools:context="niu.com.djandroid.jdroid.androidstack.MainActivity"
tools:showIn="@layout/activity_main">
<android.support.v4.widget.SwipeRefreshLayout
android:id="@+id/activity_main_swipe_refresh_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!" />
<SeekBar
android:id="@+id/seekBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="54dp" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="New Button" />
</LinearLayout>
</android.support.v4.widget.SwipeRefreshLayout>
</LinearLayout>
now in my activity i used AsyncTask
to show SwipeRefreshLayout
. also use mSwipeRefreshLayout.setOnRefreshListener(null)
to stop swipe gesture.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mSwipeRefreshLayout = (SwipeRefreshLayout) findViewById(R.id.activity_main_swipe_refresh_layout);
mSwipeRefreshLayout.setOnRefreshListener(null);
((Button) findViewById(R.id.button)).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// mSwipeRefreshLayout.setRefreshing(true);
// call AsyncTask
new LongOperation().execute("");
}
});
}
private class LongOperation extends AsyncTask<String, Void, String> {
@Override
protected String doInBackground(String... params) {
for (int i = 0; i < 5; i++) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.interrupted();
}
}
return "Executed";
}
@Override
protected void onPostExecute(String result) {
// stop mSwipeRefreshLayout
mSwipeRefreshLayout.setRefreshing(false);
}
@Override
protected void onPreExecute() {
// start mSwipeRefreshLayout
mSwipeRefreshLayout.setRefreshing(true);
}
@Override
protected void onProgressUpdate(Void... values) {
}
}
EDITED:15/02/20
After 4 year, Here is great explanation on android developer site