If you like to know how It works It's better do the following steps:
1.first of all you should know definitions of shapes
2.It's always better to consider 2D shape of them, because three dimensions may be complex for you.
so let me to explain some shapes:
Circle
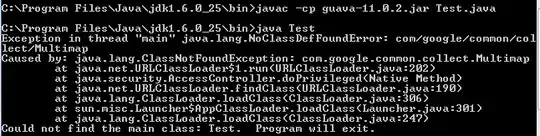
A circle is a simple closed shape. It is the set of all points in a plane that are at a given distance from a given point, the center.
You can use distance()
, length()
or sqrt()
to calculate the distance to the center of the billboard.
The book of shaders - Chapter 7
Square
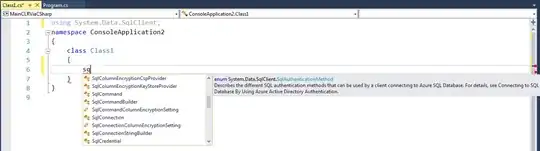
In geometry, a square is a regular quadrilateral, which means that it has four equal sides and four equal angles (90-degree angles).
I describe 2D shapes In before section now let me to describe 3D definition.
Sphere
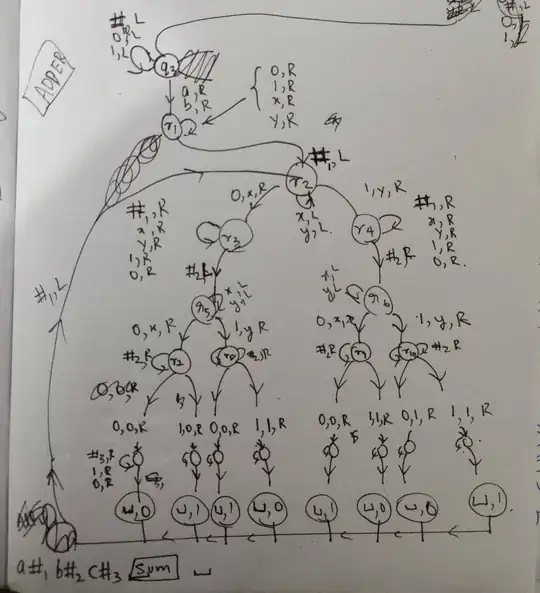
A sphere is a perfectly round geometrical object in three-dimensional space that is the surface of a completely round ball.
Like a circle, which geometrically is an object in two-dimensional space, a sphere is defined mathematically as the set of points that are all at the same distance r from a given point, but in three-dimensional space.
Refrence - Wikipedia
Cube

In geometry, a cube is a three-dimensional solid object bounded by six square faces, facets or sides, with three meeting at each vertex.
Refrence : Wikipedia
Modeling with distance functions
now It's time to understanding modeling with distance functions
Sphere
As mentioned In last sections.In below code length()
used to calculate the distance to the center of the billboard , and you can scale this shape by s parameter.
//Sphere - signed - exact
/// <param name="p">Position.</param>
/// <param name="s">Scale.</param>
float sdSphere( vec3 p, float s )
{
return length(p)-s;
}
Box
// Box - unsigned - exact
/// <param name="p">Position.</param>
/// <param name="b">Bound(Scale).</param>
float udBox( vec3 p, vec3 b )
{
return length(max(abs(p)-b,0.0));
}
length()
used like previous example.
next we have max(x,0)
It called Positive and negative parts
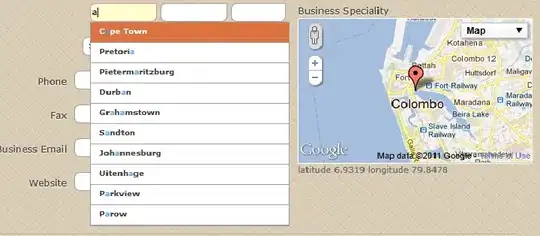
this is mean below code is equivalent:
float udBox( vec3 p, vec3 b )
{
vec3 value = abs(p)-b;
if(value.x<0.){
value.x = 0.;
}
if(value.y<0.){
value.y = 0.;
}
if(value.z<0.){
value.z = 0.;
}
return length(value);
}
step 1
if(value.x<0.){
value.x = 0.;
}
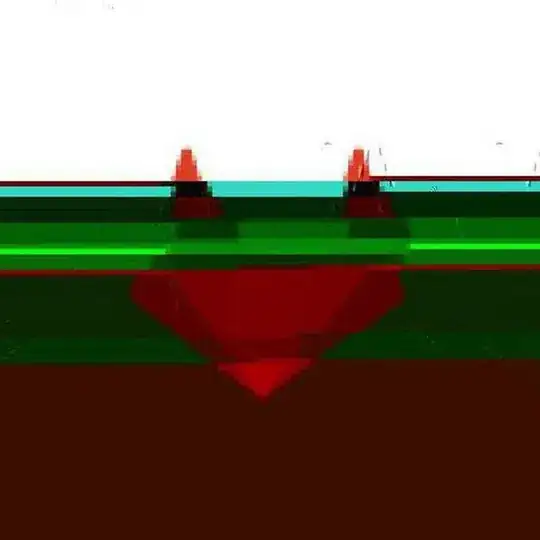
step 2
if(value.y<0.){
value.y = 0.;
}

step 3
if(value.z<0.){
value.z = 0.;
}
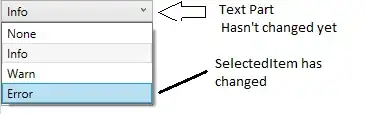
step 4
next we have absolution function.It used to remove additional parts.
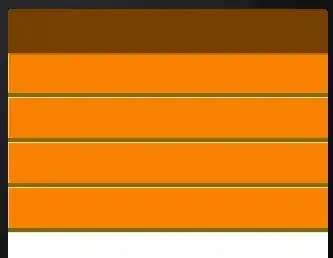
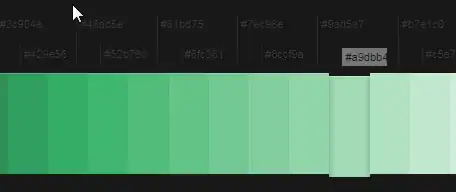
Absolution Steps
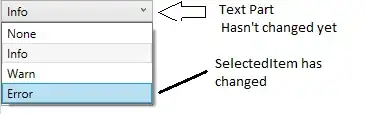
Absolution step 1
if(value.x < -1.){
value.x = 1.;
}

Absolution step 2
if(value.y < -1.){
value.y = 1.;
}
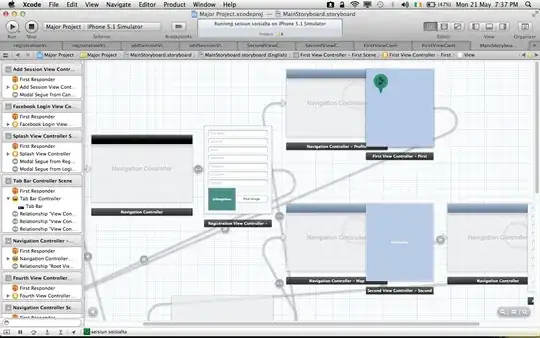
Absolution step 3
if(value.z < -1.){
value.z = 1.;
}
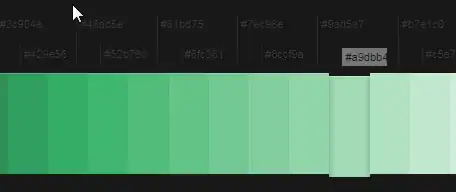
Also you can make any shape by using Constructive solid geometry.
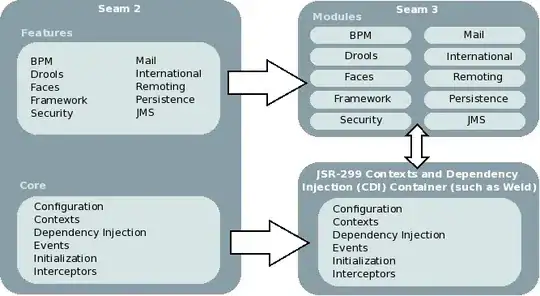
CSG is built on 3 primitive operations: intersection ( ∩
), union ( ∪
), and difference ( -
).
It turns out these operations are all concisely expressible when combining two surfaces expressed as SDFs.
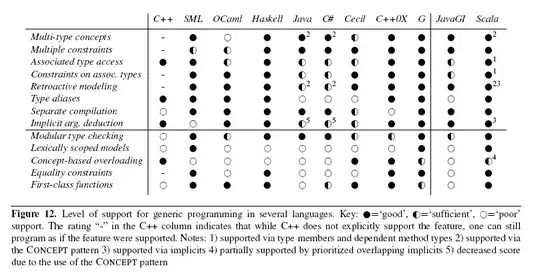
float intersectSDF(float distA, float distB) {
return max(distA, distB);
}
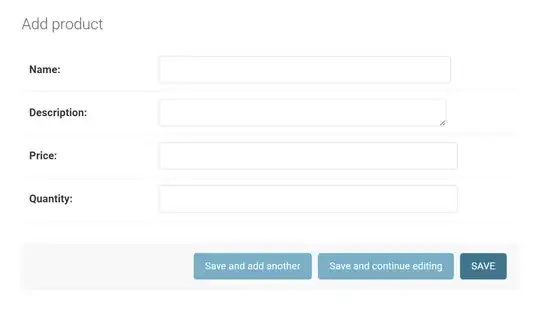
float unionSDF(float distA, float distB) {
return min(distA, distB);
}
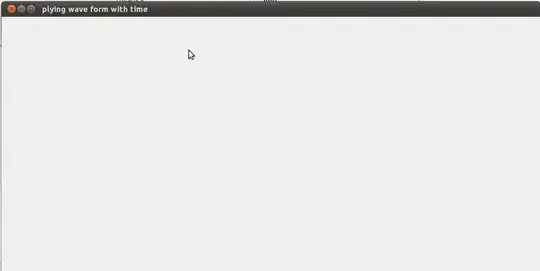
float differenceSDF(float distA, float distB) {
return max(distA, -distB);
}