Changing the font for one SKLabelNode
As you probably already know, you can change the font of a SKLabelNode
using the fontName
property.
let label = SKLabelNode(fontNamed:"Chalkduster")

label.fontName = "Arial"
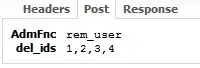
Changing the font for all the SKLabelNode(s)
Now, if I'm not wrong you want to change the fontName
for all the labels in your scene.
First of all we need an easy way to visit all the nodes in your scene graph. I mean, not only the direct children of the scene but every child of child of child...
Visiting the scene graph
The best way I can imagine is implementing a visit
extension in SKNode
extension SKNode {
func visit(logic: (node:SKNode) -> ()) {
logic(node:self)
children.forEach { $0.visit(logic) }
}
}
Now SKNode
has a visit
function that receive a closure. The closure gets applied to the current node and to every children. Then the process is recursively repeated until there are new descendants.
Launching the recursion
Now in you GameScene
you can add this code
class GameScene: SKScene {
func changeFonts() {
visit { (node) -> () in
if let label = node as? SKLabelNode {
label.fontName = "Arial"
}
}
}
}
Finally, when you're ready invoke changeFonts()
.
It should do the job.
Just remember to use a font available in SpriteKit.