You can try this :
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<android.support.v7.widget.CardView
android:id="@+id/cardView"
android:layout_margin="20dp"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:orientation="vertical"
android:layout_height="wrap_content">
<TextView
android:layout_width="match_parent"
android:text="My Title Text"
android:padding="20dp"
android:textSize="18sp"
android:textColor="#000"
android:layout_height="wrap_content" />
<TextView
android:layout_width="match_parent"
android:layout_height="2dp"
android:background="#e6b121"
android:id="@+id/your_id" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="20dp"
android:text="Lorem ipsum dolor sit amet, mel esse eripuit patrioque id. Id inimicus scripserit delicatissimi est, id vide velit erant sed. Has an veri mutat accumsan, te iusto dolore similique nec. An laboramus delicatissimi nam, ea brute consul vis."
android:id="@+id/textView17" />
</LinearLayout>
</android.support.v7.widget.CardView>
</LinearLayout>
which will give you as a result this:
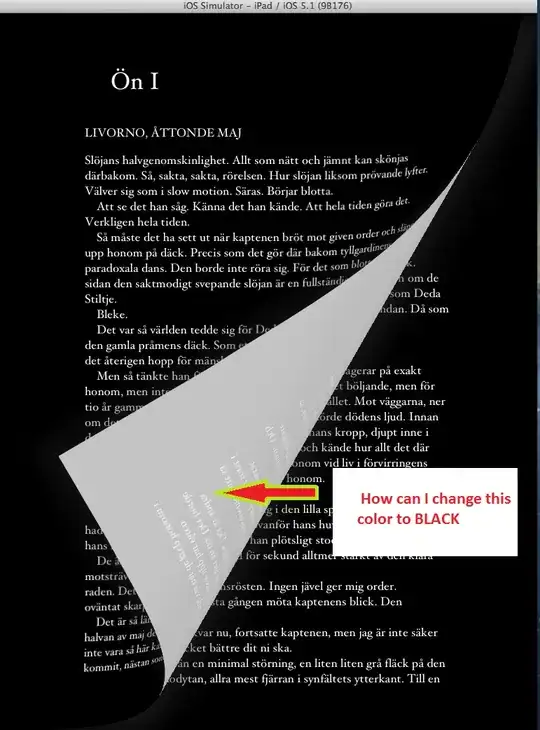
and also try this:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<android.support.v7.widget.CardView
android:id="@+id/cardView"
android:layout_margin="20dp"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:orientation="vertical"
android:layout_height="wrap_content">
<TextView
android:layout_width="match_parent"
android:text="My Title Text"
android:padding="20dp"
android:textSize="18sp"
android:textColor="#000"
android:layout_height="wrap_content" />
<TextView
android:layout_width="match_parent"
android:layout_height="2dp"
android:background="#e6b121"
android:id="@+id/your_id" />
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:padding="10dp"
android:text="Screen On:"
android:id="@+id/textView17" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:padding="10dp"
android:text="19m 28s"
android:id="@+id/textView18" />
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:padding="10dp"
android:text="Discharge while On/Off:"
android:id="@+id/textView19" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:padding="10dp"
android:text="7% / 3%"
android:id="@+id/textView20" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
</android.support.v7.widget.CardView>
</LinearLayout>
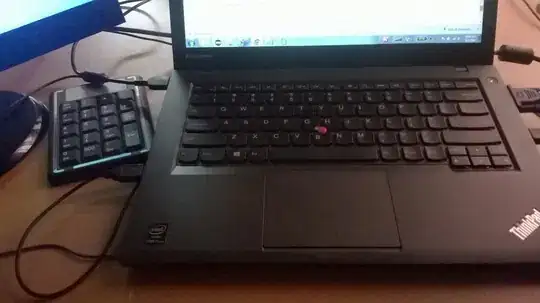
But of course you are going to need to implement a custom adapter to adapt them into a ListView
or RecyclerView
Hope it helps!!!