I build a project to test. It works, check the screenshot
iPad in Portrait
iPad in Landscape
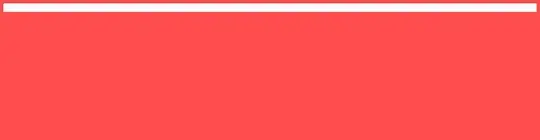
It can't be done in Storyboard, because iPad only got one layout in Storyboard.
but it's very simple to achieve what you need programmatically .
//ViewController.m
#import "ViewController.h"
@interface ViewController ()
@property (nonatomic, strong) UIView *firstView;
@property (nonatomic, strong) UIView *secondView;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
self.firstView = [[UIView alloc] initWithFrame:CGRectZero];
self.firstView.backgroundColor = [UIColor redColor];
[self.view addSubview:self.firstView];
self.secondView = [[UIView alloc] initWithFrame:CGRectZero];
self.secondView.backgroundColor = [UIColor blueColor];
[self.view addSubview:self.secondView];
[self setupSubViewLayout];
}
- (void)didRotateFromInterfaceOrientation:(UIInterfaceOrientation)fromInterfaceOrientation {
[self setupSubViewLayout];
}
- (void)setupSubViewLayout {
CGFloat viewWidth = self.view.frame.size.width;
CGFloat viewHeight = self.view.frame.size.height;
CGRect firstRect;
CGRect secondRect;
if (viewWidth > viewHeight) {
firstRect = CGRectMake(0, 0, viewWidth/2.0, viewHeight);
secondRect = CGRectMake(viewWidth/2.0, 0, viewWidth/2.0, viewHeight);
}else {
firstRect = CGRectMake(0, 0, viewWidth, viewHeight/2.0);
secondRect = CGRectMake(0, viewHeight/2.0, viewWidth, viewHeight/2.0);
}
self.firstView.frame = firstRect;
self.secondView.frame = secondRect;
}