it's not so easy, but there is a solution, you have to subclass jcombobox...
You have to subclass JComboBox
to get access to the ComboBoxUI
. To do so you set your own custom ComboBoxUI
during object instanciation (we make changes in the all constructors, see init()
in CustomComboBox
.
The ComboBoxUI
is required to get access to the ComboboxPopup
. We replace simply the default ComboboxPopup
with our custom ComboboxPopup
. You have to know that the ComboboxPopup
is responsible for the creation of the drop-down-menu, that pops up when you click on the button.
then we finally can adjust the JScrollPane
from the Popup, we grab the vertical JScrollBar
and alter its appearance (setting a custom width).
public class CustomComboBox<T> extends JComboBox<T> {
public CustomComboBox() {
super();
init();
}
public CustomComboBox(ComboBoxModel<T> aModel) {
super(aModel);
init();
}
public CustomComboBox(T[] items) {
super(items);
init();
}
public CustomComboBox(Vector<T> items) {
super(items);
init();
}
public void init(){
CustomComboBoxUI ccbui = new CustomComboBoxUI();
setUI(ccbui);
}
}
this is the custom ComboboxUI
that grants you acces to the ComboboxPopup
(quite simple):
public class CustomComboBoxUI extends BasicComboBoxUI{
protected ComboPopup createPopup() {
return new CustomComboBoxPopup( comboBox );
}
}
thankgod the custom ComboboxPopup
needs just the basic constructor overriden and only one method changed (sets the size of the scrollpan to 40px):
public class CustomComboBoxPopup extends BasicComboPopup{
public CustomComboBoxPopup(JComboBox combo) {
super(combo);
}
@Override
protected void configureScroller() {
super.configureScroller();
scroller.getVerticalScrollBar().setPreferredSize(new Dimension(40, 0));
}
}
to set the size of the combobox you simply need to adjust its size
String[] data = new String[]{"a","b","c","d","e","f","g","h","i"};
CustomComboBox<String> comboBox = new CustomComboBox(data);
comboBox.setPreferredSize(new Dimension(50,50)); //set the size you wish
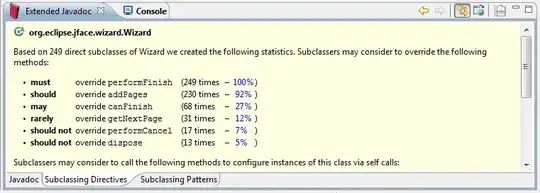
see also setting size of scroller and setting size of combobox for further help...