In principle, the below should work. I transform the points into pixel coordinates, and then use figimage to put the image at that point.
import networkx as nx
import matplotlib.pyplot as plt
import matplotlib.image as image
im = image.imread('Lower_case_a.png')
G=nx.fast_gnp_random_graph(2,1)
pos = nx.spring_layout(G)
fig = plt.figure()
ax = fig.add_subplot(111)
ax.patch.set_alpha(0)
ax.axis(xmin=-1,xmax=2,ymin=-1,ymax=2)
for node in G.nodes():
x,y = pos[node]
trans_x, trans_y = ax.transData.transform((x,y))
fig.figimage(im,trans_x,trans_y) #could use custom image for each node
nx.draw_networkx_edges(G,pos)
plt.savefig('tmp.png')
It almost works for me. I get:
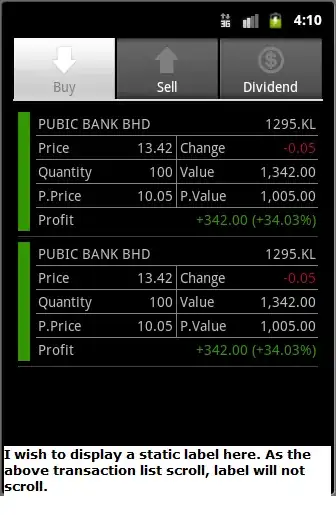
I think that's because of some weird issue on my computer. When I run the code provided at matplotlib.org to explain transData.transform, it should give me
, but instead I get 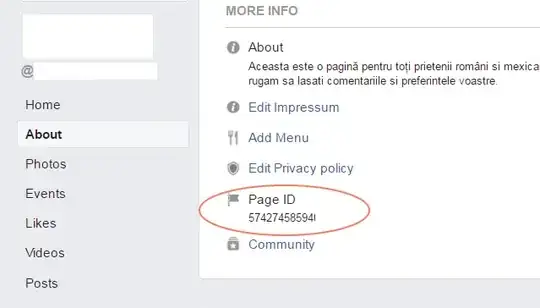
So I feel like the offset I'm seeing may be a problem on my machine and I'd like to try another computer. At any rate, let me know if this works for you and hopefully it at least points you in the right direction.