I have made a very simple layout with a listview. I add a button to the listview items and listen to the click to insert a new object before the button.
public MainWindow()
{
InitializeComponent();
listViewtst.Items.Add("test");
listViewtst.Items.Add("test2");
Button btn = new Button();
btn.Content = "+";
btn.Click += Btn_Click;
listViewtst.Items.Add(btn);
}
private void Btn_Click(object sender, RoutedEventArgs e)
{
listViewtst.Items.Insert(listViewtst.Items.Count - 1, "added by the btn");
}
Not sure if it is the best solution but this is how i would do it if no one showed me a better way :)
Other solution:
Code behind
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
private ObservableCollection<MyObject> _windows = new ObservableCollection<MyObject>();
public MainWindow()
{
InitializeComponent();
Windows.Add(new MyObject { Title = "Collection Item 1" });
Windows.Add(new MyObject { Title = "Collection Item 2" });
}
private void Btn_Click(object sender, RoutedEventArgs e)
{
}
public ObservableCollection<MyObject> Windows
{
get { return _windows; }
set { _windows = value; }
}
private void button_Click(object sender, RoutedEventArgs e)
{
Windows.Add(new MyObject { Title = "From Btn" });
}
}
public class MyObject
{
public string Title { get; set; }
}
Xaml:
<Window x:Class="Move_To_Prd_Dev.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="246" Width="325" Name="UI">
<Window.Resources>
<CollectionViewSource Source="{Binding ElementName=UI, Path=Windows}" x:Key="YourMenuItems"/>
</Window.Resources>
<Grid DataContext="{Binding ElementName=UI}">
<ListBox x:Name="listTxt" HorizontalAlignment="Left" Height="163" Margin="10,29,0,0" VerticalAlignment="Top" Width="297">
<ListBox.ItemsSource>
<CompositeCollection>
<CollectionContainer Collection="{Binding Source={StaticResource YourMenuItems}}" />
<Button x:Name="button" Content="Button" HorizontalAlignment="Left" VerticalAlignment="Top" Width="75" Click="button_Click"/>
</CompositeCollection>
</ListBox.ItemsSource>
</ListBox>
</Grid>
This uses CompositeCollection to add the button control to the list (with the advantage that the button is always Last)
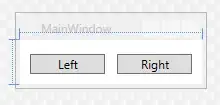