Rails is all about convention over configuration. Meaning if you write your Rails applications following Rails conventions, you'll have huge benefit from it.
Your tables should be named in plural form. Tables and Posts. What I understood from your comments is that user has many posts and posts can belong to many users. This is typical has_and_belongs_to_many association.
If you rename your saved_post table as posts_users (both table names in alphabetical order) Rails will know how to deal with these tables automatically.
Database should look like this:
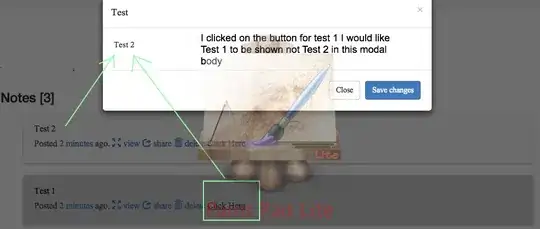
I made a sample application with your two user and methods to add posts to either of those users.
# routes.rb
root 'posts#index'
resources :posts
# posts_controller.rb
class PostsController < ApplicationController
before_action :set_user
def index
@posts = @current_user.posts
end
def new
@post = @current_user.posts.build(title: 'totally new title')
end
def edit
@post = Post.find(params[:id])
end
def create
@post = Post.new(post_params)
@post.save
redirect_to posts_path
end
def update
@post = Post.find(params[:id])
if @post.update(post_params)
redirect_to posts_path
else
render 'edit'
end
end
# --------------------------------------------------------------------------
private
# --------------------------------------------------------------------------
# Sets the user based on the params value
def set_user
if params[:user_id]
@current_user = User.find(params[:user_id])
else
# If not found, sets the first from the Users table
@current_user = User.first
end
end
def post_params
params.require(:post).permit(:title, {user_ids: []})
end
end
index
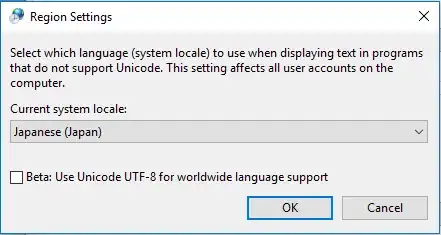
# index.html.erb
<h3>Users</h3>
<% User.all.each do |user| %>
<%= link_to user.username, posts_path(user_id: user.id) %> <br />
<% end %>
<h3>Posts for the user: <%= @current_user.username %></h3>
<p>
<% @posts.each do |post| %>
<%= link_to post.title, edit_post_path(post) %> <br />
<% end %>
</p>
<p>
<%= link_to 'Create new post', new_post_path %>
</p>
In edit you can choose which users are attached to this post:
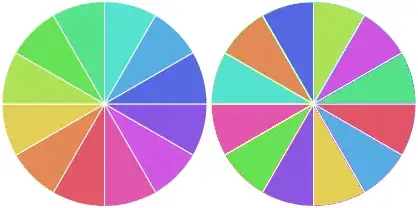
# edit.html.erb
<%= render :partial => "post_form", locals: {button_name: 'update'} %>
# _post_form.html.erb
<h3>Post <%= @post.title %> for the user <%= @current_user.username %></h3>
<%= form_for @post do |f| %>
<%= f.text_field :title %> <br />
<p>
<label>
<%= f.collection_check_boxes(:user_ids, User.all, :id, :username) %>
<label><br />
</p>
<p>
<%= f.submit button_name, class: "btn btn-default btn-save" %>
</p>
<% end %>
In post.rb and user.rb files you need to specify association between these two classes. And when you have posts_users table named correctly, Rails finds it automatically.
# post.rb
class Post < ActiveRecord::Base
has_and_belongs_to_many :users
end
# user.rb
class User < ActiveRecord::Base
has_and_belongs_to_many :posts
end
And if you create a new title, if will use the same form:

# new.html.erb
<%= render :partial => "post_form", locals: {button_name: 'create'} %>