Using a slightly modified version of the code in this other answer
I have the following code:
class ViewController: UIViewController {
@IBOutlet weak var webView: UIWebView!
lazy var documentsPath = {
return NSSearchPathForDirectoriesInDomains(.DocumentDirectory, .UserDomainMask, true)[0]
}()
let fileName = "file.pdf"
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
createPDF()
loadPDF()
}
func createPDF() {
let html = "<b>Hello <i>World!</i></b> <p>Generate PDF file from HTML in Swift</p>"
let fmt = UIMarkupTextPrintFormatter(markupText: html)
// 2. Assign print formatter to UIPrintPageRenderer
let render = UIPrintPageRenderer()
render.addPrintFormatter(fmt, startingAtPageAtIndex: 0)
// 3. Assign paperRect and printableRect
let page = CGRect(x: 0, y: 0, width: 595.2, height: 841.8) // A4, 72 dpi
let printable = CGRectInset(page, 0, 0)
render.setValue(NSValue(CGRect: page), forKey: "paperRect")
render.setValue(NSValue(CGRect: printable), forKey: "printableRect")
// 4. Create PDF context and draw
let pdfData = NSMutableData()
UIGraphicsBeginPDFContextToData(pdfData, CGRectZero, nil)
for i in 1...render.numberOfPages() {
UIGraphicsBeginPDFPage();
let bounds = UIGraphicsGetPDFContextBounds()
render.drawPageAtIndex(i - 1, inRect: bounds)
}
UIGraphicsEndPDFContext();
// 5. Save PDF file
pdfData.writeToFile("\(documentsPath)/\(fileName)", atomically: true)
}
func loadPDF() {
let filePath = "\(documentsPath)/\(fileName)"
let url = NSURL(fileURLWithPath: filePath)
let urlRequest = NSURLRequest(URL: url)
webView.loadRequest(urlRequest)
}
}
This code works, it creates a PDF file and then loads the same PDF file into a webView. The only thing that I changed was to create a lazy variable that returns the documents directory and I use a constant for the file path.
You should be able to use the same methods to save and retrieve your PDF file.
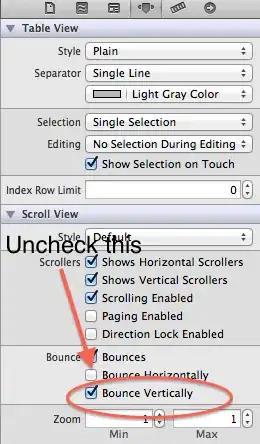