The link you've presented brings a world map. The example I'm showing here only has the markers with lines connecting them (so they are not geo-localized). In any case using matplotlib (don't know about GNUplot) you can adapt the following recipe:
import random
import matplotlib.pyplot as plt
x = ['01.01.1960','12.01.1960','12.01.1960','01.01.1970','13.04.1980']
y = ['Heavy Rain','Sunshine','Slight Hail','Heavy Rain','Slight Hail']
l = ['New York','Sacramento','Seattle','Boston','San Francisco']
nx,ny = [],[]
for i in range(len(x)):
nx.append(i)
ny.append(-i)
s = random.randint(100,150)
m = random.choice(['o','s','^','d'])
color = random.randint(0,255)/255,random.randint(0,255)/255,random.randint(0,255)/255
plt.scatter(i,-i,s=s,marker=m,color=color,label=y[i])
plt.text(i,-i,x[i])
plt.plot(nx,ny,'--')
plt.legend()
plt.show()
Which will give an image like this:
Notice I'm changing the size of the marker, the marker itself, color, adding text to each point, and in the end prompting a legend.
If you want to draw a map considering real locations you might want to take a look at Basemap
EDIT (after poster clearing it's intention):
The following code:
xt = [63072000,64022400,64022400,315532800,639964800,706320000]
y2 = [33,12,21,33,21,33]
l = ['New York','Sacramento','Seattle','Boston','San Francisco','Seattle']
lm = ['o','s','^','d','*','^']
cl = ['red','blue','green','orange','purple','green']
import matplotlib.pyplot as plt
from matplotlib.dates import YearLocator, MonthLocator, DateFormatter,AutoDateLocator
import datetime
# get the dates into something readable
x2 = [datetime.datetime.fromtimestamp(i) for i in xt]
years = YearLocator() # every year
months = MonthLocator() # every month
yearsFmt = DateFormatter('%Y')
auto = AutoDateLocator()
# plot lines and markers
fig, ax = plt.subplots()
ax.plot_date(x2, y2, '--',color='black')
for i in range(len(x2)):
ax.scatter(x2[i],y2[i],s=300,marker=lm[i],color=cl[i])
plt.text(x2[i],y2[i],l[i])
# format the ticks
ax.xaxis.set_major_locator(auto)
ax.xaxis.set_major_formatter(yearsFmt)
ax.xaxis.set_minor_locator(months)
ax.autoscale_view()
ax.set_yticks([10,20,30], minor=False)
ax.set_yticklabels(['Sunny','More or Less','Rainy'])
#ax.yticks([10,20,30], ['Sunny','More or Less','Rainy'], rotation='vertical')
ax.fmt_xdata = DateFormatter('%Y-%m-%d')
ax.grid(True)
fig.autofmt_xdate()
plt.show()
, will result in the following result:
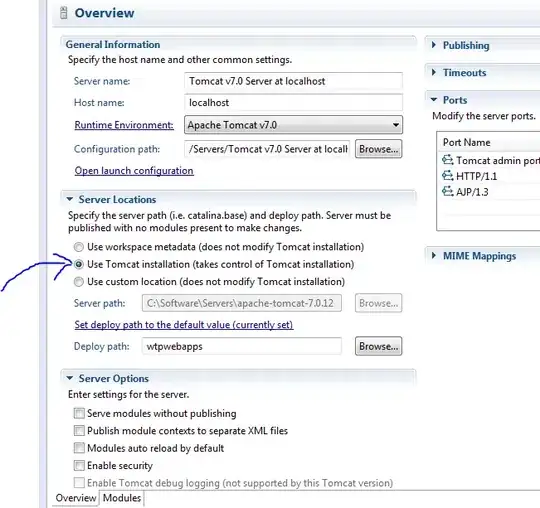
NOTE: I must say this is a really strange plot. Intuitively it seems to me the trend should for each city and not between cities (you should have a line for each city, although you don't seem to have the data to do such a plot). In any case this is the code that does your request.