Following the comment by roadrunner66 I assembled an example that works for me
l, = plt.plot(x,y+5)
l.set_dashes([5,2])
plt.axis(xmin=0,xmax=5,ymin=100,ymax=150)
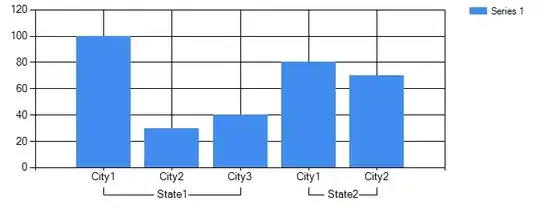
If you want to automate this, I guess one needs to know the length of your line as well in order to calculate the right dashes.
To obtain the length of the line in display coordinates could do something like
xl,yl=l.get_xydata().T
xmin, xmax = ax.get_xlim()
ymin, ymax = ax.get_ylim()
# Filter out the points outside the range & replace them with a point on the axis
m = ((xl >= xmin) & (xl <= xmax)) & ((yl >= ymin) & (yl <= ymax))
x = np.concatenate([[xmin], xl[m], [xmax]])
y = np.concatenate([[ymin], yl[m], [ymax]])
xt, yt = ax.transData.transform(np.vstack([xl,yl]).T).T
length = np.sum( np.sqrt((xt[1:] - xt[:-1])**2 + (yt[1:] - yt[:-1])**2))
Now given this length we can calculate a reasonable tick length & spacing in between
length = np.sum( np.sqrt((x[1:] - x[:-1])**2 + (y[1:] - y[:-1])**2))
tickl = 5.
minspace = 2.
i = int(length / (tickl + minspace))
space = length / i - tickl # since length = i * (space + tickl)
print space
This gives me a value of 2.39086935951
so 2 wasn't a too bad guess.