First off, know that the connection and command variables need to be properly disposed of. We ensure this by using a using
block like so:
using (MySqlConnection conn = new MySqlConnection())
using (MySqlCommand cmd = new MySqlCommand()) {
// ensure your connection is set
conn.ConnectionString = "your connection string";
cmd.Connection = conn;
}
Then, work up your CommandText
using @ParamName
to denote parameters:
using (MySqlConnection conn = new MySqlConnection())
using (MySqlCommand cmd = new MySqlCommand()) {
// ensure your connection is set
conn.ConnectionString = "your connection string";
cmd.Connection = conn;
cmd.CommandText = "INSERT INTO ThisTable (Field1, Field2) VALUES (@Param1, @Param2);";
}
Next, use cmd.Parameters.AddWithValue() to insert your values. This should be done so the framework can avoid SQL Injection attacks (Rustam's answer is very dangerous, as is the way you were doing it):
using (MySqlConnection conn = new MySqlConnection())
using (MySqlCommand cmd = new MySqlCommand()) {
// ensure your connection is set
conn.ConnectionString = "your connection string";
cmd.Connection = conn;
cmd.CommandText = "INSERT INTO ThisTable (Field1, Field2) VALUES (@Param1, @Param2);";
cmd.Parameters.AddWithValue("@Param1", userAdmin.YourProperty);
cmd.Parameters.AddWithValue("@Param2", userAdmin.YourOtherProperty);
}
and finally, you can then open the connection and execute it:
using (MySqlConnection conn = new MySqlConnection())
using (MySqlCommand cmd = new MySqlCommand()) {
// ensure your connection is set
conn.ConnectionString = "your connection string";
cmd.Connection = conn;
cmd.CommandText = "INSERT INTO ThisTable (Field1, Field2) VALUES (@Param1, @Param2);";
cmd.Parameters.AddWithValue("@Param1", userAdmin.YourProperty);
cmd.Parameters.AddWithValue("@Param2", userAdmin.YourOtherProperty);
cmd.Connection.Open();
cmd.ExecuteNonQuery();
cmd.Connection.Close();
}
And finally, realize that when these command and connection variables exit the using
block, they will be properly disposed of. The using
block ensures proper disposal regardless of any exceptions that might be thrown or whether we forget to close/dispose ourselves.
Now, for helpful debugging, wrap the core execution and connection opening in a try/catch
block, and write the exception to the output window if there is one:
using (MySqlConnection conn = new MySqlConnection())
using (MySqlCommand cmd = new MySqlCommand()) {
// ensure your connection is set
conn.ConnectionString = "your connection string";
cmd.Connection = conn;
cmd.CommandText = "INSERT INTO ThisTable (Field1, Field2) VALUES (@Param1, @Param2);";
cmd.Parameters.AddWithValue("@Param1", userAdmin.YourProperty);
cmd.Parameters.AddWithValue("@Param2", userAdmin.YourOtherProperty);
try {
cmd.Connection.Open();
cmd.ExecuteNonQuery();
cmd.Connection.Close();
} catch (Exception e) {
System.Diagnostics.Debug.WriteLine("EXCEPTION: " + e.ToString());
}
}
At this point you set a breakpoint on the exception catching and check your locals window to see what your state is... in locals, you should see your cmd
variable... expand it, look for the Parameters
collection, expand that, check the count and values, etc, as shown:
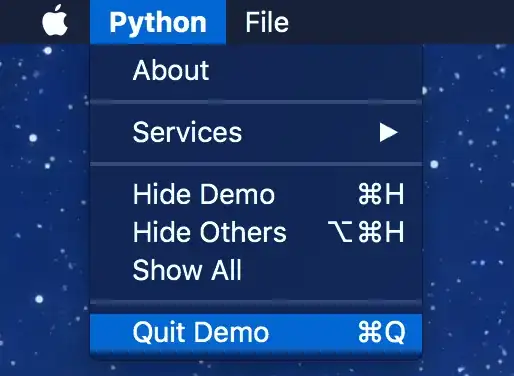