Summary of the Solution: I would use a Grid
layout with automatically expanding ColumnDefinitions
(the default setting of Width="1*"
. When the button is clicked and disappears (Use Opacity="0"
), you should change the width of the corresponding ColumnDefinition
to 0
.
Here are the before and after screen shots of what you want:
Before
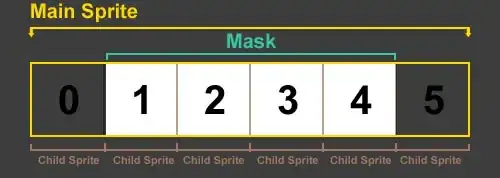
After Click Donkey Button
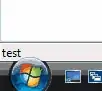
After Click Bird Button
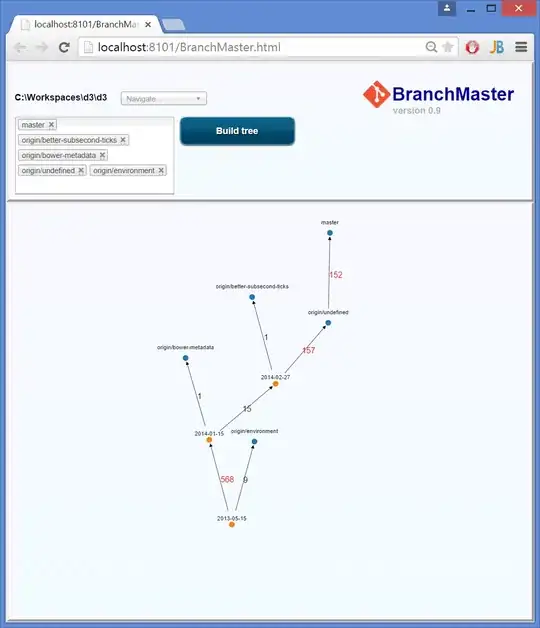
After Click Moose Button
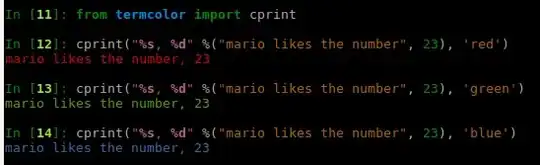
After Click Giraffe Button(I accidentally resized before I screenshotted)
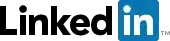
I know that you are using MVVM, but this problem is entirely in the user interface, so its encouraged to use code-behind for this solution. I don't recommend putting this type of code into mvvm.
For this solution, use a grid:
<Window x:Class="WpfApplication4.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApplication4"
mc:Ignorable="d"
Title="Used for question 36258073" >
<Grid>
<Grid.Resources>
<Style TargetType="Button">
<EventSetter Event="Click" Handler="AllButton_Click"/>
<Setter Property="Margin" Value="5"/>
<Setter Property="HorizontalContentAlignment" Value="Center"/>
</Style>
</Grid.Resources>
<Grid.ColumnDefinitions>
<ColumnDefinition Name="ColumnDefinitionNull"/>
<ColumnDefinition Name="ColumnDefinitionOne"/>
<ColumnDefinition Name="ColumnDefinitionTwo"/>
<ColumnDefinition Name="ColumnDefinitionThree"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Button Name="ButtonNull" Content="Test1 Giraffe" Grid.Row="0" Grid.Column="0" />
<Button Name="ButtonOne" Content="Test2 Bird" Grid.Row="0" Grid.Column="1" />
<Button Name="ButtonTwo" Content="Test3 Donkey" Grid.Row="0" Grid.Column="2" />
<Button Name="ButtonThree" Content="Test4 Moose" Grid.Row="0" Grid.Column="3" />
</Grid>
and the code-behind for the button click is:
private void AllButton_Click(object sender, RoutedEventArgs e)
{
Button button = (Button)sender;
button.Opacity = 0;
var zeroWidth = new GridLength(0.0);
switch (button.Name)
{
case "ButtonNull":
ColumnDefinitionNull.Width = zeroWidth;
break;
case "ButtonOne":
ColumnDefinitionOne.Width = zeroWidth;
break;
case "ButtonTwo":
ColumnDefinitionTwo.Width = zeroWidth;
break;
default:
ColumnDefinitionThree.Width = zeroWidth;
break;
}
}
The idea for the Opacity 0 came from another Stack Overflow post here. The notion about the buttons stretching in place of the other button disappearring comes from button layout and grid definition ideas this Stack Overflow post1, and this Stack Overflow post2, and this Stack Overflow post3 and this last related Stack Overflow post4.