Without modifing class A
code you won't be able to UT the ReadBlock
method using Moq. You'll be able to UT this method using code weaving tools (MsFakes, Typemock Isolator, etc...)
For example(MsFakes):
[TestMethod]
public void TestMethod1()
{
using (ShimsContext.Create())
{
ShimCloudBlockBlob.AllInstances.<the method you want to override> = (<the method arguments>) => {};
}
}
Inside the using
scope you'll be able to override any method CloudBlockBlob has, through the property AllInstances
.
In the next section I'm going to discuss all the other options you have...
Option 1:
public class A
{
private IBlockBlob _blockBlob;
public A(IBlockBlob blockBlob)
{
_blockBlob = blockBlob;
}
public void ReadBlock()
{
_blockBlob.DoSomething();
}
}
Since you create a new instance each time call ReadBlock
(your method's current behavior) you better inject a factory instead of wrapper and DoSomething
should be create
; Option 2:
public class A
{
private readonly IFactoryBlockBlob _blobFctory;
public A(IFactoryBlockBlob blobFctory)
{
_blobFctory = blobFctory;
}
public void ReadBlock()
{
var blob = _blobFctory.Create();
}
}
However, based on your question and your comments it seems that your class 'has a dependency' instead of 'needs a dependency'.
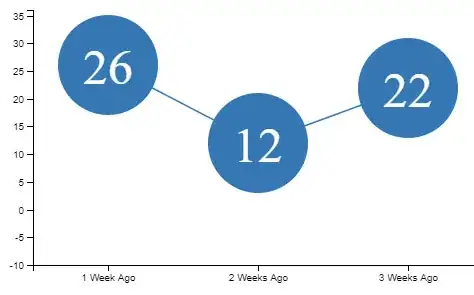
(Mark Siemens wrote a great book about DI, this chart was taken from his book)
With this new piece of information your method should be something like; Option 3:
public class A
{
public void ReadBlock(ICloudBlob blob)
{
}
}
But you don't want to change the signature of the method:
public class A
{
public void ReadBlock()
{
ReadBlock(new CloudBlockBlob(<the params bla bla...>));
}
internal void ReadBlock(ICloudBlob blob)
{
}
}
Add InternalsVisibleToAttribute
, then verify the behavior of the internal method.
By reading between the lines, it feels to me that your class is a kind of "legacy code" meaning that it can do the job, won't change, and verifying its behavior might be a waste of time. In the past I've posted a chart (in this answer) which may help you to decide the way to handle this case.