I wrote the answer, which you used as an example, many years ago. Now I would just to place the subgrid data together with the main data of the row, like details
property below:
var myGridData = [
// main grid data
{id: "10", col1: "11", col2: "12", details: [
// data for subgrid for the id=10
{id: "10", c1: "aa", c2: "ab", c3: "ac"},
{id: "20", c1: "ba", c2: "bb", c3: "bc"},
{id: "30", c1: "ca", c2: "cb", c3: "cc"}
]},
{id: "20", col1: "21", col2: "22", details: [
// data for subgrid for the id=20
{id: "10", c1: "xx", c2: "xy", c3: "xz"}
]}
];
The expression $(this).jqGrid("getLocalRow", rowid)
get the item of the data and $(this).jqGrid("getLocalRow", rowid).details
is the subgrid data of the row. As the result we can rewrite the original example like on the demo.
To have the column with the fixed text Details
we can use simple formatter
formatter: function () {
return details;
}
where details
is defined for example like
var details = "<span class='fa fa-fw fa-plus'></span> " +
"<span class='mylink'>Details</span>";
(I used Font Awesome icon) and class mylink
defined like
.mylink { text-decoration: underline; }
Now we can hide the "subgrid" column and to open/close the subgrid by simulation of click
event on the hidden cell with +
or -
icon. We receive the following full code
var myGridData = [
// main grid data
{id: "10", col1: "11", col2: "12", details: [
// data for subgrid for the id=10
{id: "10", c1: "aa", c2: "ab", c3: "ac"},
{id: "20", c1: "ba", c2: "bb", c3: "bc"},
{id: "30", c1: "ca", c2: "cb", c3: "cc"}
]},
{id: "20", col1: "21", col2: "22", details: [
// data for subgrid for the id=20
{id: "10", c1: "xx", c2: "xy", c3: "xz"}
]}
],
$grid = $("#list"),
details = "<span class='fa fa-fw fa-plus'></span> " +
"<span class='mylink'>Details</span>";
$grid.jqGrid({
data: myGridData,
colModel: [
{ name: "col1", label: "Column 1" },
{ name: "col2", label: "Column 2" },
{ name: "details", label: "Details",
align: "center", width: 70,
formatter: function () {
return details;
} }
],
cmTemplate: { width: 200 },
iconSet: "fontAwesome",
autoencode: true,
sortname: "col1",
sortorder: "desc",
pager: true,
caption: "Demonstrate how to create subgrid from local data",
beforeSelectRow: function (rowid, e) {
var $self = $(this),
p = $self.jqGrid("getGridParam"),
$td = $(e.target).closest("tr.jqgrow>td"),
cm = $td.length > 0 ? p.colModel[$td[0].cellIndex] : null,
cmName = cm != null ? cm.name : null;
if (cmName === "details") {
// simulate opening the subgrid
$($td.parent()[0].cells[p.iColByName.subgrid]).click();
// inverse +/-
var $plusMinus = $td.find("span.fa");
if ($plusMinus.hasClass("fa-minus")) {
$plusMinus.removeClass("fa-minus").addClass("fa-plus");
} else {
$plusMinus.removeClass("fa-plus").addClass("fa-minus");
}
}
return true;
},
subGrid: true,
subGridRowExpanded: function (subgridDivId, rowid) {
var $subgrid = $("<table id='" + subgridDivId + "_t'></table>"),
$subgridDiv = $("#" + subgridDivId),
subgridData = $(this).jqGrid("getLocalRow", rowid).details;
$subgridDiv.closest(".subgrid-data").prev(".subgrid-cell").remove();
var colspan = $subgridDiv.closest(".subgrid-data").attr("colspan");
$subgridDiv.closest(".subgrid-data").attr("colspan", parseInt(colspan, 10) + 1);
$subgridDiv.append($subgrid);
$subgrid.jqGrid({
idPrefix: rowid + "_",
data: subgridData,
colModel: [
{ name: "c1", label: "Col 1" },
{ name: "c2", label: "Col 2" },
{ name: "c3", label: "Col 3" }
],
iconSet: "fontAwesome",
autowidth: true,
autoencode: true,
sortname: "c1"
});
$subgrid.jqGrid("setGridWidth", $subgridDiv.width() - 1);
}
}).jqGrid("hideCol", "subgrid");
The corresponding demo one can see here. After clicking of "+ Detailes" one will see the following:
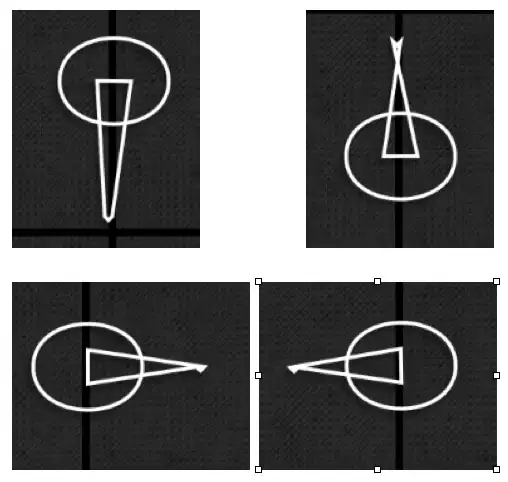