I was able to achieve this simple example
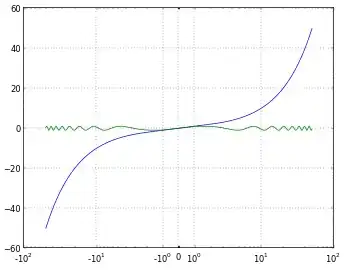
by using a subclass of UINavigationBar and setting a collection view to the view controller's navigationItem.titleView
#import <UIKit/UIKit.h>
@interface NavigationBar : UINavigationBar
@end
#import "NavigationBar.h"
@implementation NavigationBar
- (CGSize)sizeThatFits:(CGSize)size
{
return CGSizeMake(self.superview.bounds.size.width, 80);
}
-(void)setFrame:(CGRect)frame {
CGRect f = frame;
f.size.height = 80;
[super setFrame:f];
}
@end
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
@end
#import "ViewController.h"
#import "CollectionViewCell.h"
@interface ViewController ()<UICollectionViewDelegate, UICollectionViewDataSource>
@property (nonatomic, weak) UICollectionView *collectionView;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
UICollectionViewFlowLayout *layout=[[UICollectionViewFlowLayout alloc] init];
UICollectionView *collectionView = [[UICollectionView alloc] initWithFrame:CGRectMake(0, 0, 300, 80) collectionViewLayout:layout];
collectionView.backgroundColor = [UIColor clearColor];
self.navigationItem.titleView = collectionView;
[collectionView registerClass:[CollectionViewCell class] forCellWithReuseIdentifier:@"cell"];
collectionView.delegate = self;
collectionView.dataSource = self;
}
-(NSInteger)numberOfSectionsInCollectionView:(UICollectionView *)collectionView
{
return 1;
}
-(NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section
{
return 5;
}
-(UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath
{
CollectionViewCell *cell = (CollectionViewCell *)[collectionView dequeueReusableCellWithReuseIdentifier:@"cell" forIndexPath:indexPath];
cell.backgroundColor = [UIColor orangeColor];
return cell;
}
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout*)collectionViewLayout sizeForItemAtIndexPath:(NSIndexPath *)indexPath
{
return CGSizeMake(50, 50);
}
@end
I set the custom NavigationBar in the Interface Builder
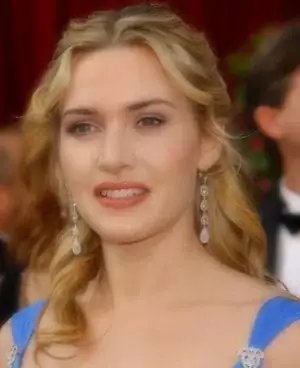
Disclaimer 1: I cannot promise that this is a future proof solution or will work in any case. I didn't test it with things like auto layout or rotation.
Disclaimer 2: I personally would implement any datasource/delegate independently from the view controller. For tableViews and collection views I use my self grown OFAPopulator.
Here is a example code: gitlab.com/vikingosegundo/collectionview-in-navigationbar/tree/… there are still some UI quirks in it. But probably that was to be expected. I guess Apple just did not consider us changing the height. Actually I never saw an app with a different height for it's nav bar. But the code answers your question. Give it more love and it may be useful for you.