Image storage must be performed in some directory and the corresponding paths of the image must be stored in the database.
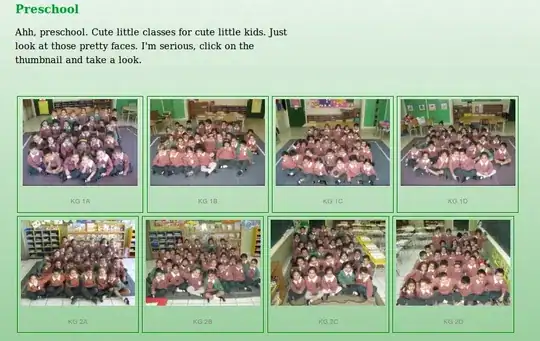
There will be times when you will accessing your images from one acivity then the other, in that case you will just need to pass the path of the image from activity one to activity two and then retrieve the image from the directory to display in activity two.
Image storing and loading from databases may turn out to be a pain when the size of the images will start increasing.
For learning how to store images, Give an eye to this
CODE EXAMPLE
private void SaveImage(Bitmap finalBitmap) {
String root = Environment.getExternalStorageDirectory().toString();
File myDir = new File(root + "/saved_images");
myDir.mkdirs();
Random generator = new Random();
int n = 10000;
n = generator.nextInt(n);
String fname = "Image-"+ n +".jpg";
File file = new File (myDir, fname);
if (file.exists ()) file.delete ();
try {
FileOutputStream out = new FileOutputStream(file);
finalBitmap.compress(Bitmap.CompressFormat.JPEG, 90, out);
out.flush();
out.close();
} catch (Exception e) {
e.printStackTrace();
}
}
In the above code, the line
Environment.getExternalStorageDirectory()
is refering to android/data folder. you can create folder inside upto any level, like android/data/folderone/folderTwo/folderThree
.
Note: However you need to first fetch the images from server for the first time and store them in device.If you are thinking of bundling up the images along with the app, put all of your images in res/drawable folders.(if no web server functionality is involved)