The date-time API of java.util
and their formatting API, SimpleDateFormat
are outdated and error-prone. It is recommended to stop using them completely and switch to the modern date-time API. For any reason, if you have to stick to Java 6 or Java 7, you can use ThreeTen-Backport which backports most of the java.time functionality to Java 6 & 7. If you are working for an Android project and your Android API level is still not compliant with Java-8, check Java 8+ APIs available through desugaring and How to use ThreeTenABP in Android Project.
In order to stick to English
, you need to specify Locale.ENGLISH
with the date-time formatting/parsing API. Anyway, one of the best practices is to use Locale
with the formatting/parsing API in every case.
Modern API:
DateTimeFormatter dtf = DateTimeFormatter.ofPattern("HH:mm:ss", Locale.ENGLISH);
Legacy API:
SimpleDateFormat sdf = new SimpleDateFormat("HH:mm:ss", Locale.ENGLISH);
Note that the java.util.Date
object is not a real date-time object like the modern date-time types; rather, it represents the milliseconds from the Epoch of January 1, 1970
. When you print an object of java.util.Date
, its toString
method returns the date-time calculated from this milliseconds value. Since java.util.Date
does not have timezone information, it applies the timezone of your JVM and displays the same. If you need to print the date-time in a different timezone, you will need to set the timezone to SimpleDateFomrat
and obtain the formatted string from it.
In contrast, modern date-time API has specific classes representing just date, or time or date-time. And, for each of these, there are separate classes for with/without timezone information. Check out the following table for an overview of modern date-time types:
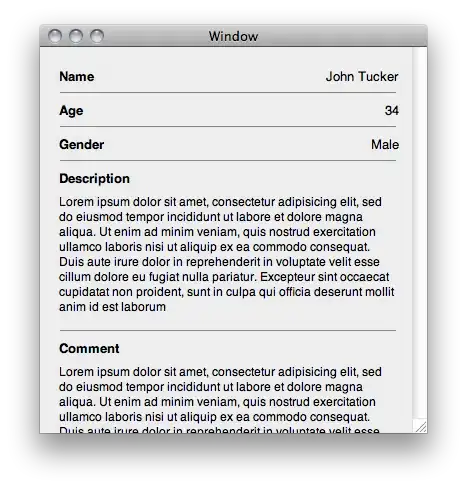
A quick demo:
import java.time.LocalTime;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class Main {
public static void main(String[] args) {
LocalTime time = LocalTime.now(ZoneId.of("Europe/London"));
// Print its default format i.e. the value returned by time#toString
System.out.println(time);
// Custom format
DateTimeFormatter dtf = DateTimeFormatter.ofPattern("hh:mm:ss", Locale.ENGLISH);
System.out.println(time.format(dtf));
}
}
Output:
12:39:07.627763
12:39:07
Learn more about the modern date-time API from Trail: Date Time.