Unfortunately, no - there is no such property.
This is a rather difficult thing to do since you need to measure all rows before you can figure out which one has the widest text. There is not a cross-platform way to do that. There are ways to do it at the platform level for Android and iOS, but I don't think there is a way for WinPhone.
[edited to reflect a better approach identified in the comments]:
An alternative is to use OneWayToSource
bindings and keeping track of the ColumnDefinition
entries for each listview row.
XamlPage.Xaml:
<?xml version="1.0" encoding="UTF-8"?>
<ContentPage
xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="FormsSandbox.XamlPage">
<ContentPage.Padding>
<OnPlatform x:TypeArguments="Thickness" iOS="0,20,0,0" Android="0" WinPhone="0"/>
</ContentPage.Padding>
<AbsoluteLayout>
<ListView x:Name="MyLV" AbsoluteLayout.LayoutBounds="0,0,1,1" AbsoluteLayout.LayoutFlags="All">
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<Grid RowSpacing="0" ColumnSpacing="0">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Label Grid.Column="0" Text="{Binding .}" MinimumWidthRequest="50" HorizontalOptions="StartAndExpand" SizeChanged="LabelSizeChanged" />
<Label Grid.Column="1" Text="Second Column"/>
</Grid>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</AbsoluteLayout>
</ContentPage>
XamlPage.xaml.cs:
using System;
using Xamarin.Forms;
using System.Collections.Generic;
namespace FormsSandbox
{
public partial class XamlPage : ContentPage
{
private double _colSize = 0.0;
private List<ColumnDefinition> _columns = new List<ColumnDefinition>();
public XamlPage ()
{
InitializeComponent ();
var data = new List<string> ();
data.Add ("Lorem ipsum");
data.Add ("Foo");
data.Add ("Dolor semet");
data.Add ("Test");
data.Add (".");
data.Add ("Xamarin Forms Is Great");
data.Add ("Short");
data.Add ("Longer than Short");
data.Add ("");
data.Add ("Hyphenated");
data.Add ("Non-hyphenated");
data.Add ("Ironic, eh?");
MyLV.ItemsSource = data;
}
public void LabelSizeChanged (object sender, EventArgs e)
{
var label = (Label)sender;
var grid = (Grid)label.Parent;
var column = grid.ColumnDefinitions [0];
if (!_columns.Contains (column)) {
_columns.Add (column);
}
var adjustments = new List<ColumnDefinition> ();
if (label.Width > _colSize) {
_colSize = label.Width;
adjustments.AddRange (_columns);
} else {
adjustments.Add (column);
}
foreach (var col in adjustments) {
col.Width = new GridLength (_colSize);
}
}
}
}
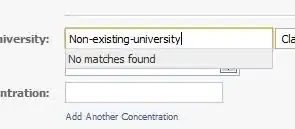