I combined this JLayeredPane
example with this CardLayout
example to demonstrate multiple, selectable layered panes.
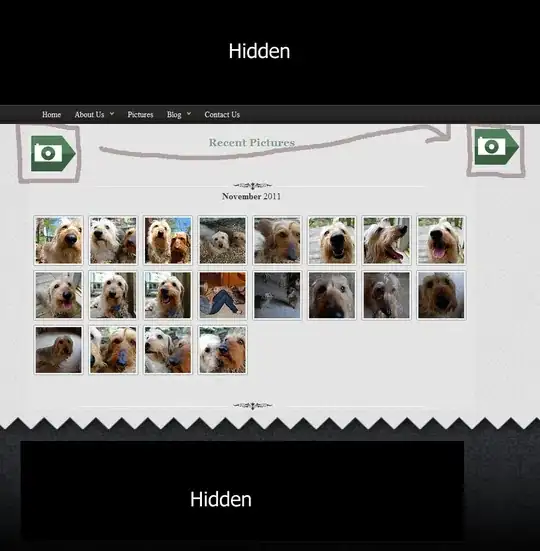
import java.awt.BorderLayout;
import java.awt.CardLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.RenderingHints;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLayeredPane;
import javax.swing.JPanel;
/**
* @see https://stackoverflow.com/a/36587584/230513
* @see https://stackoverflow.com/a/6432291/230513
* @see https://stackoverflow.com/questions/6432170
*/
public class CardPanel extends JLayeredPane {
private static final Dimension d = new Dimension(320, 240);
private static final Random random = new Random();
private static final JPanel cards = new JPanel(new CardLayout());
private static final JComboBox combo = new JComboBox();
private final String name;
public CardPanel(String name) {
this.name = name;
this.setBackground(new Color(random.nextInt()));
this.add(new LayerPanel(1 * d.height / 8), 100);
this.add(new LayerPanel(2 * d.height / 8), 101);
this.add(new LayerPanel(3 * d.height / 8), 102);
}
@Override
public Dimension getPreferredSize() {
return d;
}
@Override
public String toString() {
return name;
}
private static class LayerPanel extends JPanel {
private static final Random r = new Random();
private int n;
private Color color = new Color(r.nextInt());
public LayerPanel(int n) {
this.n = n;
this.setOpaque(false);
this.setBounds(n, n, d.width / 2, d.height / 2);
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
g2d.setRenderingHint(
RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
g2d.setColor(color);
g2d.fillRoundRect(0, 0, getWidth(), getHeight(), 16, 16);
g2d.setColor(Color.black);
g2d.drawString(String.valueOf(n), 5, getHeight() - 5);
}
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
create();
}
});
}
private static void create() {
JFrame f = new JFrame();
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
for (int i = 1; i < 9; i++) {
CardPanel p = new CardPanel("Panel " + String.valueOf(i));
combo.addItem(p);
cards.add(p, p.toString());
}
JPanel control = new JPanel();
combo.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JComboBox jcb = (JComboBox) e.getSource();
CardLayout cl = (CardLayout) cards.getLayout();
cl.show(cards, jcb.getSelectedItem().toString());
}
});
control.add(combo);
f.add(cards, BorderLayout.CENTER);
f.add(control, BorderLayout.SOUTH);
f.pack();
f.setLocationRelativeTo(null);
f.setVisible(true);
}
}