You cannot do serious layouts withe GridLayout
or BoxLayout
. Forget
about them.
The first question is: Are you sure you don't want the JTextArea
and JTextField
to grow? It is a standard in UI that these components are growing and shrinking; the first one in both directions and the second one horizontally.
Anyway, I post solutions for GroupLayout
and MigLayout
, which are
the managers I highly recommend.
a) Solution with GroupLayout
Each component has three sizes: minimum, preferred, and maximum. These
are set to some sensible defaults for each component. This is why the
JTextField
tends to grow horizontally; its maximum value is set to a
very large number.
package com.zetcode;
import java.awt.Container;
import java.awt.EventQueue;
import javax.swing.BorderFactory;
import javax.swing.GroupLayout;
import javax.swing.JComponent;
import javax.swing.JFrame;
import static javax.swing.JFrame.EXIT_ON_CLOSE;
import javax.swing.JLabel;
import javax.swing.JTextArea;
import javax.swing.JTextField;
public class GroupLayoutStretchingEx extends JFrame {
public GroupLayoutStretchingEx() {
initUI();
}
private void initUI() {
JTextArea area = new JTextArea(12, 20);
area.setBorder(BorderFactory.createEtchedBorder());
JLabel input = new JLabel("Input");
JTextField textField = new JTextField(15);
createLayout(area, input, textField);
setTitle("Stretching");
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
}
private void createLayout(JComponent... arg) {
Container pane = getContentPane();
GroupLayout gl = new GroupLayout(pane);
pane.setLayout(gl);
gl.setAutoCreateContainerGaps(true);
gl.setAutoCreateGaps(true);
gl.setHorizontalGroup(gl.createParallelGroup(GroupLayout.Alignment.TRAILING)
.addComponent(arg[0], GroupLayout.DEFAULT_SIZE,
GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addGroup(gl.createSequentialGroup()
.addComponent(arg[1])
.addComponent(arg[2], GroupLayout.DEFAULT_SIZE,
GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
);
gl.setVerticalGroup(gl.createSequentialGroup()
.addComponent(arg[0], GroupLayout.DEFAULT_SIZE,
GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addGroup(gl.createParallelGroup()
.addComponent(arg[1])
.addComponent(arg[2], GroupLayout.DEFAULT_SIZE,
GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
);
pack();
}
public static void main(String[] args) {
EventQueue.invokeLater(() -> {
GroupLayoutStretchingEx ex = new GroupLayoutStretchingEx();
ex.setVisible(true);
});
}
}
In GroupLayout
, you need to set the correct values for the maximum
component size in the addComponent()
method.
JTextArea area = new JTextArea(12, 20);
By setting the number of rows and columns for the JTextArea
, we
set a preffered size for this component. This value is later used
by the layout manager in its calculations.
b) Solution with MigLayout
In MigLayout
, we control the resizement of the components with various
constraints, such as fill
, grow
, or push
. By not using them, the
components do not grow.
package com.zetcode;
import javax.swing.BorderFactory;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.SwingUtilities;
import net.miginfocom.swing.MigLayout;
public class MigLayoutStretchingEx extends JFrame {
public MigLayoutStretchingEx() {
initUI();
}
private void initUI() {
JTextArea area = new JTextArea(12, 20);
area.setBorder(BorderFactory.createEtchedBorder());
JLabel input = new JLabel("Input");
JTextField textField = new JTextField(15);
createLayout(area, input, textField);
setTitle("MigLayout example");
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private void createLayout(JComponent... arg) {
setLayout(new MigLayout());
add(arg[0], "wrap");
add(arg[1], "split 2");
add(arg[2], "growx");
pack();
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
MigLayoutStretchingEx ex = new MigLayoutStretchingEx();
ex.setVisible(true);
});
}
}
And here is the screenshot:
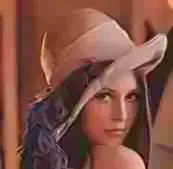