Edit: Add solution by using .plist file
One way, you can store the information into a .plist file.
- Press command+N to add a new .plist file with name of UserInformation.plist (You can replace UserInformation with any name you like.) and edit it as below
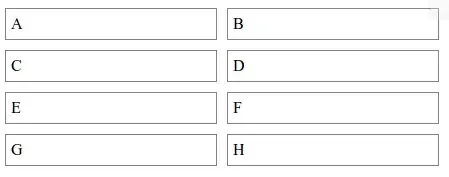
Then you can store user name into a array.
if let path = NSBundle.mainBundle().pathForResource("UserInformation", ofType: "plist") {
if let data = NSArray(contentsOfFile: path) {
let userName = NSMutableArray.init(capacity: 1)
for dict in data {
userName.addObject(dict.valueForKey("User")!)
}
// Now user names are stored into a array
}
}
Another way, you can also store the information into a local .json file though JSON is usually used in communication between client and server.
Form iOS5, Apple provided APIs to support parse JSON.
Use any text editor you like to create a json file with content below and save it with name UserInfo.json (You can replace UserInfo with any name you like.)
[
{ "User":"asf", "FirstName":"john", "LastName":"smith"},
{ "User":"adfkh", "FirstName":"carrie", "LastName":"underwood"}
]
Add it to your project.
Now you can store the user name into a array.
if let path = NSBundle.mainBundle().pathForResource("UserInfo", ofType: "json") {
if let data = NSData(contentsOfFile: path) {
do {
let jsonData = try NSJSONSerialization.JSONObjectWithData(data, options:NSJSONReadingOptions.MutableContainers ) as! NSMutableArray
let userName = NSMutableArray.init(capacity: 1);
for dict in jsonData {
userName.addObject(dict.valueForKey("User")!)
}
// Now user names are stored into a array
} catch {
// report error
}
}
}
For more details about JSON, you can visit www.json.org.