Just like you did, just write down that formula:
expr = 'D * sin(C * atan(B*x - E*(B* x - atan(B*x)))) + A';
ft = fittype(expr, 'independent', 'x');
opts = fitoptions('Method', 'NonlinearLeastSquares');
opts.StartPoint = ones(1,5); % [A,B,C,D,E]
[fitresult, gof] = fit(x, y, ft, opts)
plot(fitresult, x, y)
EDIT:
Based on the Wikipedia article, here's a small example:
% some data based on equation
B = 0.714;
C = 1.4;
D = 800;
E = -0.2;
f = @(x) D * sin(C * atan(B*(1-E)*x + E*atan(B*x)));
x = linspace(0,10,200)'; %'
y = f(x);
% add noise
yy = y + randn(size(x))*16;
% fit
%expr = 'D * sin(C * atan(B*(1-E)*x + E*atan(B*x)))';
expr = 'D * sin(C * atan(B*x - E*(B* x - atan(B*x))))';
ft = fittype(expr, 'independent', 'x', 'dependent','y');
opts = fitoptions('Method', 'NonlinearLeastSquares');
opts.StartPoint = [1 1 1000 1]; % [B C D E]
[fitresult, gof] = fit(x, y, ft, opts)
% plot
yhat = feval(fitresult, x);
h = plot(x,y,'b-', x,yhat,'r-', x,yy,'g.');
set(h, 'LineWidth',2)
legend({'y', 'yhat', 'y+noise'}, 'Location','SouthEast')
grid on
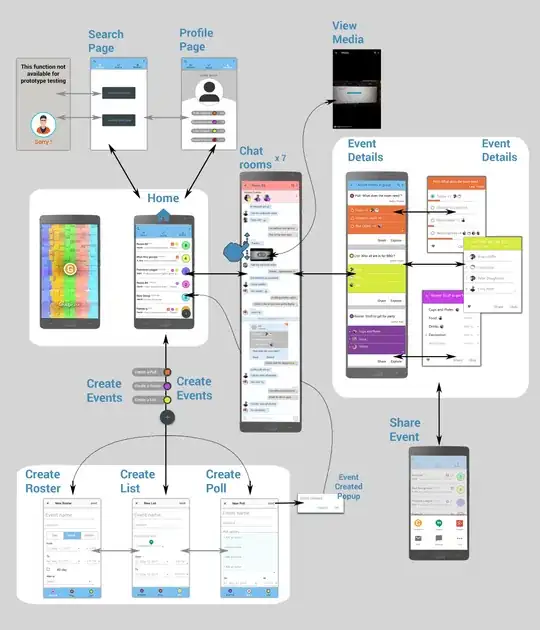
Result:
fitresult =
General model:
fitresult(x) = D * sin(C * atan(B*x - E*(B* x - atan(B*x))))
Coefficients (with 95% confidence bounds):
B = 0.5916 (0.5269, 0.6563)
C = 1.899 (1.71, 2.089)
D = 783.7 (770.5, 796.9)
E = 1.172 (1.136, 1.207)
gof =
sse: 6.6568e+04
rsquare: 0.9834
dfe: 196
adjrsquare: 0.9832
rmse: 18.4291
Note that using a starting point like [1 1 1 1]
is far from the true solution, so fitting will stop without converging (the scale of D
parameter is very different from the other params B
, C
, and E
)... Instead I had to start somewhere closer [1 1 1000 1]
.