Normally when you use a navigation controller to wrap the view controller, TopLayoutGuide
(and BottomLayoutGuide
will be set for you and with "Adjust Scroll View Insets" that you can set in the storyboard file, it will automatically set contentInsets
of the outermost scrollview for you to accommodate that. But since you don't use a navigation controller to wrap your view controller here, you have like three options.
1. Manually set the frame of the table view to offset for the status bar's height.
We just move the table view down 20 points and make it 20 points shorter. The ideal place is somewhere in your code we know it will be called every time the superview's bound is changed. One of them is in viewDidLayoutSubviews
.
Try putting this code in your view controller's code.
override func viewDidLayoutSubviews() {
super.viewDidLayoutSubviews()
self.view.superview!.backgroundColor = UIColor.whiteColor()
let insets = UIEdgeInsets(top: 20, left: 0, bottom: 0, right: 0)
self.view.frame = UIEdgeInsetsInsetRect(self.view.superview!.bounds, insets)
}
Please note that I have to set the background color of the superview's to white because the superview (which is likely to be UIWindow
) might have no background color and it will make the top part appears black. And normally in the production code, you might want to check whether the superview's bound has really changed before setting the frame.
2. Use UIViewController
and add UITableView
as subview.
This way Interface Builder will allow you to set the constraints all you want.
3. Just wrap this with UINavigationController
And it will appear like most apps that have a navigation bar on the top. This might not be what you want. But I rarely see a table view without wrapping by a navigation controller.
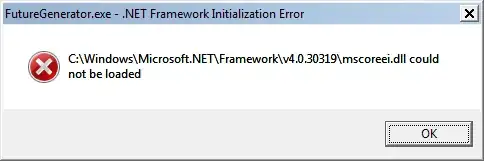