This is how I would do. Pleas note that this is a single class solution for example purpose, you can separate classes
- This will keep only 5 fragments at a time
- Screen is divided into 4 buttons you can set alpha value of buttons and size as per need currently i kept it half transparent for example
- I am using
Glide
for image loading to avoid OOM problem because of image loading
What it look like
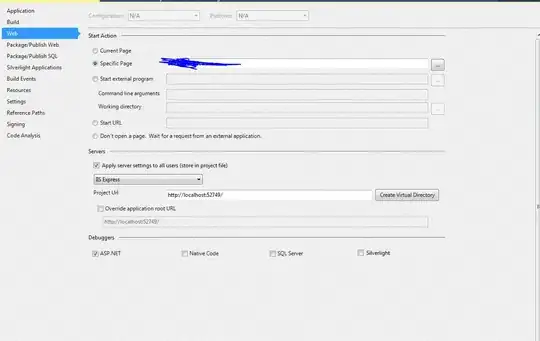
The Solution :-
package com.example.sample;
import java.util.ArrayList;
import com.bumptech.glide.Glide;
import android.content.Context;
import android.media.Ringtone;
import android.media.RingtoneManager;
import android.net.Uri;
import android.os.Bundle;
import android.os.Parcelable;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentActivity;
import android.support.v4.view.PagerAdapter;
import android.support.v4.view.ViewPager;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.view.View.OnClickListener;
import android.widget.FrameLayout;
import android.widget.ImageView;
public class MainActivity extends FragmentActivity {
private static ArrayList<Integer> imageList = new ArrayList<Integer>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Load image data
for (int i = 0; i < 70; i++) {
imageList.add(R.drawable.ic_launcher);
}
if (savedInstanceState == null) {
getSupportFragmentManager().beginTransaction()
.add(R.id.container, new PlaceholderFragment()).commit();
}
}
/**
* A placeholder fragment containing a simple view.
*/
public static class PlaceholderFragment extends Fragment {
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_main, container,
false);
ViewPager viewPager = (ViewPager) rootView
.findViewById(R.id.image_pager);
// Limit number of pages that should be retained to either
// side of the current page
viewPager.setOffscreenPageLimit(2);
viewPager.setAdapter(new SongDetailAdapter(getActivity()));
return rootView;
}
}
public static class SongDetailAdapter extends PagerAdapter {
Context mContext;
LayoutInflater mLayoutInflater;
public SongDetailAdapter(Context context) {
mContext = context;
mLayoutInflater = (LayoutInflater) mContext
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
}
@Override
public int getCount() {
return imageList.size();
}
@Override
public boolean isViewFromObject(View view, Object object) {
return view == ((FrameLayout) object);
}
@Override
public Object instantiateItem(ViewGroup container, final int position) {
View itemView = mLayoutInflater.inflate(
R.layout.image_place_holder, container, false);
ImageView imageView = (ImageView) itemView
.findViewById(R.id.background);
itemView.findViewById(R.id.button1).setOnClickListener(
new OnClickListener() {
@Override
public void onClick(View v) {
playSound(1);
}
});
itemView.findViewById(R.id.button2).setOnClickListener(
new OnClickListener() {
@Override
public void onClick(View v) {
playSound(2);
}
});
itemView.findViewById(R.id.button3).setOnClickListener(
new OnClickListener() {
@Override
public void onClick(View v) {
playSound(3);
}
});
itemView.findViewById(R.id.button4).setOnClickListener(
new OnClickListener() {
@Override
public void onClick(View v) {
playSound(4);
}
});
Glide.with(mContext).load("").placeholder(imageList.get(position))
.crossFade(300).into(imageView);
container.addView(itemView);
return itemView;
}
@Override
public void destroyItem(ViewGroup container, int position, Object object) {
container.removeView((FrameLayout) object);
}
@Override
public Object instantiateItem(View arg0, int arg1) {
// TODO Auto-generated method stub
return null;
}
@Override
public Parcelable saveState() {
// TODO Auto-generated method stub
return null;
}
/*
* Play sound
*/
private void playSound(int buttonNumber) {
switch (buttonNumber) {
case 1: // play sound for Button1
case 2: // play sound for Button2
case 3: // play sound for Button3
case 4: // play sound for Button4
default: // play sound for Button n
// Playing default notification here for example
Uri notification = RingtoneManager
.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION);
Ringtone r = RingtoneManager
.getRingtone(mContext, notification);
r.play();
break;
}
}
}
}
Layouts
Main Activity layout activity_main.xml
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.sample.MainActivity"
tools:ignore="MergeRootFrame" />
Main fragment layout fragment_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.sample.MainActivity$PlaceholderFragment" >
<android.support.v4.view.ViewPager
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/image_pager"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</RelativeLayout>
Image place holder with dummy buttons imae_place_holder.xml
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<ImageView
android:id="@+id/background"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1.0"
android:orientation="horizontal" >
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1.0"
android:alpha=".5"
android:background="@android:color/white"
android:text="Button" />
<Button
android:id="@+id/button2"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1.0"
android:alpha=".5"
android:background="@android:color/holo_green_light"
android:text="Button" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1.0"
android:orientation="horizontal" >
<Button
android:id="@+id/button3"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1.0"
android:alpha=".5"
android:background="@android:color/holo_blue_light"
android:text="Button" />
<Button
android:id="@+id/button4"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1.0"
android:alpha=".5"
android:background="@android:color/holo_red_light"
android:text="Button" />
</LinearLayout>
</LinearLayout>
</FrameLayout>