As I've seen times and times again - simply mentioning eval
makes people instantly upset, even if it was a proper use case (which this is not).
So I'm going to go with another hate magnet - parsing nested structures with regexes.
Iteration (1) - a naive approach:
JSON.parse(myvar.gsub(/=>/, ':'))
Problem - will mess up your data if the string key/values contain =>
.
Iteration (2) - even number of "
s remaining mean you are not inside a string:
JSON.parse(myvar.gsub(/=>(?=(?:[^"]*"){2}*[^"]*$)/, ':'))
Problem - there might be a "
inside a string, that is escaped with a slash.
Iteration (3) - like iteration (2), but count only "
that are preceded by unescaped slashes. An unescaped slash would be a sequence of odd number of slashes:
eq_gt_finder = /(?<non_quote>
(?:
[^"\\]|
\\{2}*\\.
)*
){0}
=>(?=
(?:
\g<non_quote>
"
\g<non_quote>
){2}*
$
)/x
JSON.parse(myvar.gsub(eq_gt_finder, ':'))
See it in action
Q: Are you an infallible divine creature that is absolutely certain this will work 100% of the time?
A: Nope.
Q: Isn't this slow and unreadable as shit?
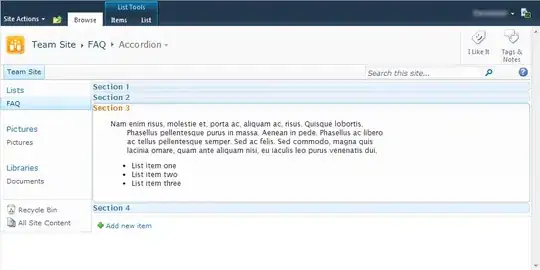
Q: Ok?
A: Yep.