Suppose you have a bunch of latitude, longitude geocoordinates.
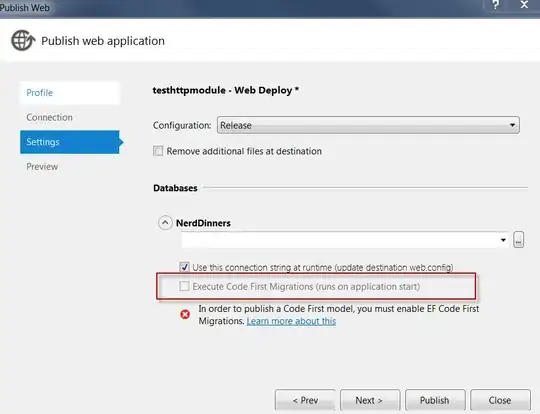
If you want to calculate the density of the bounding box that fits around your geocoordinates, then make one O(N) pass through your data set and determine the geocoordinates of the corners.
Once you find them, use the Haversine formula (Java implementation here) to calculate the length of the edges between two corners. Be sure to consistently choose either miles or kilometers for your unit of distance. After you compute the edge distances, you can compute the box's area in units of km^2 or miles^2. From there, compute the density as number of points divided by the area.
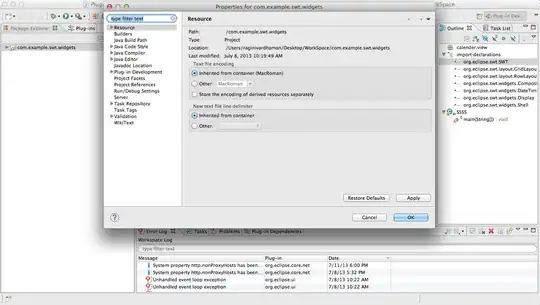
If you want to make an ad hoc query for the density around a single target point, then choose a radius R in miles or km. Make one O(N) pass through the data set, and compute the Haversine distance between your target point and every other point. If the other point is within distance R to your target, then add it to a result list. Then compute the density as the number of points within the circle defined by the radius.
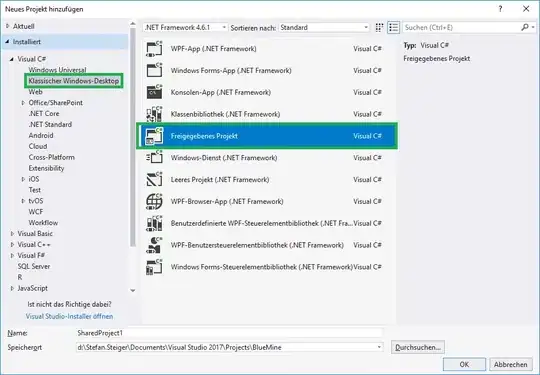
If you make a lot of these types of queries, then precompute a spatial indexing data structure. Popular indexes are R-Trees, R*-Trees, and k-d Trees. Below is a picture of an R-Tree from Wikipedia. The tree decomposes the space into rectangular regions so you can query for points quickly.
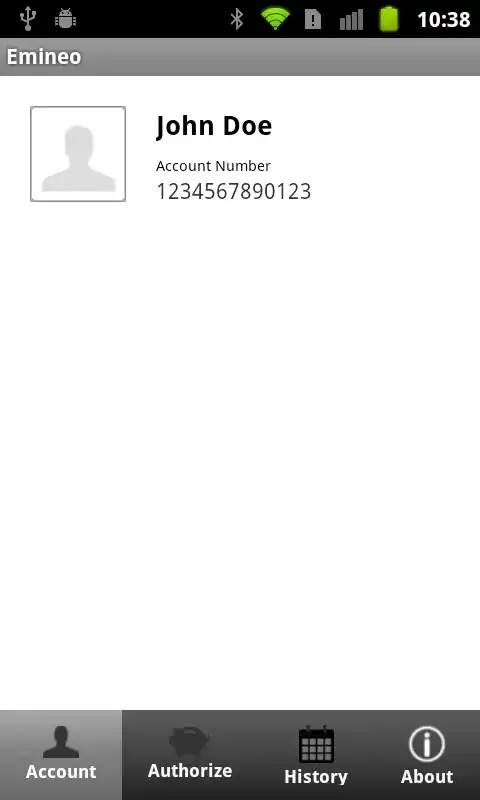
If your points can fit into memory, then use an open-source library that implements one of these data structures. Here is a link to one library called rtree that I found that allows you to find all points within some radius. I have not personally used that library.
If your points do not fit into memory, then you can use an SQL database. For example, Oracle Spatial implements these types of data structures.