Note About Alternation
In alternation, every alternative is checked at current position in the string until one of the alternation succeeds or all of them fails.
Case I
Your string is
water watering
Your regex is
/\b(water|watering)\b/g
i) First of all, first alternation is checked like \bwater
. It succeeds and water
is matched because there is a space after water in water watering
which serves as end word boundary.
ii) Due to g
flag again a match is performed. So string watering
is being tried to match with \bwater\b
(along with word boundary in end) but it fails because there is i
in watering after water
which is not word boundary. Then second alternation is checked i.e. \bwatering
and it succeeds because there is end of string which serves as word boundary for \bwatering\b
in last.
Case II
Your string is
water water-ing
For regex
/\b(water|water-ing)\b/g
i) Same as Step I of Case I
Now string upto water is consumed and our checking position is blank space before watering
water water-ing
^^
||
ii) Again a checking is performed due to g
flag. First alternation is tried with \bwater
. The position now is -
just after r
and before i
water water-ing
^^
||
Quoting from here about word boundary
A word boundary, in most regex dialects, is a position between \w and
\W (non-word char), or at the beginning or end of a string if it
begins or ends (respectively) with a word character ( [0-9A-Za-z_] ).
The dash is not a word character.
So -
acts as a word boundary and \bwater\b
is matched in water-ing
Case III
For regex
/\b(water-ing|water)\b/g
i) First alternation \bwater-ing
is checked in the string but it does not matches the string water
. Again, second alternation \bwater
is checked and it succeeds because there is a space after water
in the string.
ii) First alternation \bwater-ing
is checked in the string which is present. The string ends with this word water-ing
. So end of string($
) acts as word boundary. and match succeeds.
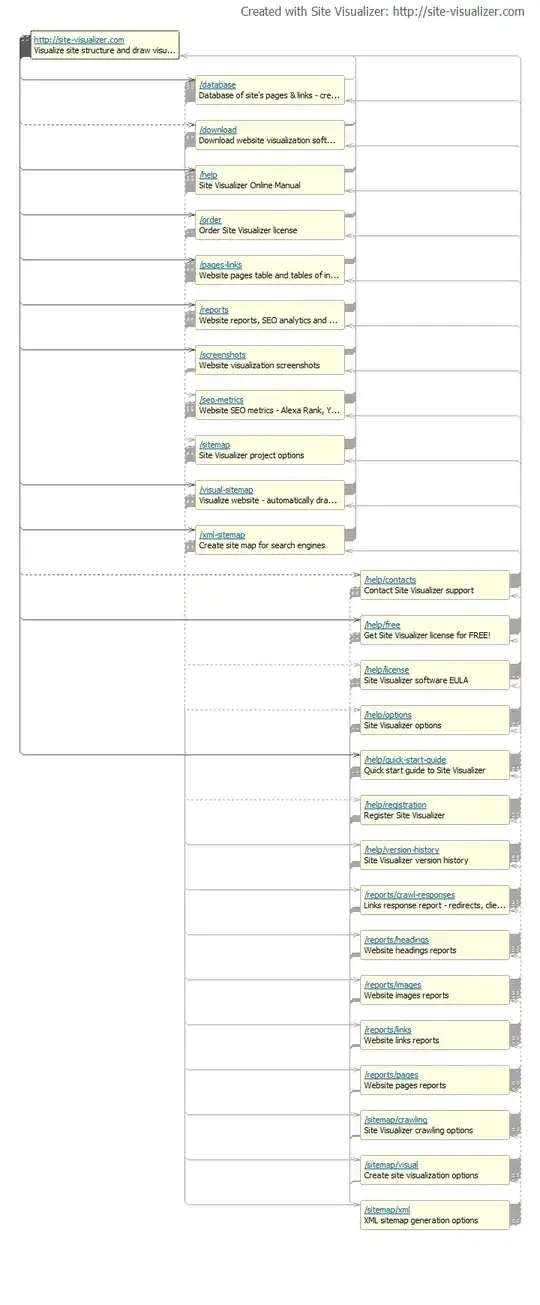
What's the solution?
i) If there is overlapping regex, keep the longest one in starting and so on as you used in your last solution
ii) You can use negative lookahead like
\b(water(?!-)|water-ing)\b
It seems Wiktor has already suggested four solutions. You can use any of them